NumPy: eye() function
eye() function
The eye() function is used to create a 2-D array with ones on the diagonal and zeros elsewhere.
Syntax:
numpy.eye(N, M=None, k=0, dtype=<class 'float'>, order='C')
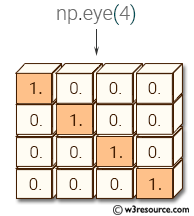
Version: 1.15.0
Parameter:
Name | Description | Required / Optional |
---|---|---|
N | Number of rows in the output. | Required |
M | Number of columns in the output. If None, defaults to N. | optional |
k | Index of the diagonal: 0 (the default) refers to the main diagonal, a positive value refers to an upper diagonal, and a negative value to a lower diagonal. | optional |
dtype | Data-type of the returned array. | optional |
order | Whether the output should be stored in row-major (C-style) or column-major (Fortran-style) order in memory | optional |
Return value:
[ndarray of shape (N,M)] An array where all elements are equal to zero, except for the k-th diagonal, whose values are equal to one.
Example: NumPy.eye()
>>> import numpy as np
>>> np.eye(2)
array([[ 1., 0.],
[ 0., 1.]])
>>> np.eye(2,3)
array([[ 1., 0., 0.],
[ 0., 1., 0.]])
>>> np.eye(3, 3)
array([[ 1., 0., 0.],
[ 0., 1., 0.],
[ 0., 0., 1.]])
>>>
Pictorial Presentation:
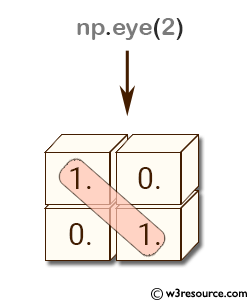
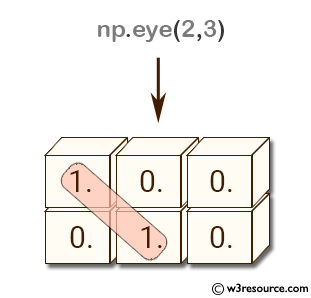

Example: NumPy.eye() where data type is int
>>> import numpy as np
>>> np.eye(2, dtype=int)
array([[1, 0],
[0, 1]])
>>> np.eye(2,2, dtype=int)
array([[1, 0],
[0, 1]])
>>> np.eye(2,2, dtype=float)
array([[ 1., 0.],
[ 0., 1.]])
>>>
Python - NumPy Code Editor:
Previous: empty_like()
Next: identity()
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework