NumPy: empty() function
empty() function
The empty() function is used to create a new array of given shape and type, without initializing entries.
Syntax:
numpy.empty(shape, dtype=float, order='C')
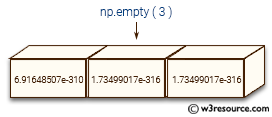
Version: 1.15.0
Parameter:
Name | Description | Required / Optional |
---|---|---|
shape | Shape of the empty array, e.g., (2, 3) or 2. | Required |
dtype | Desired output data-type for the array, e.g, numpy.int8. Default is numpy.float64. | optional |
order | Whether to store multi-dimensional data in row-major (C-style) or column-major (Fortran-style) order in memory. | optional |
Return value:
[ndarray] Array of uninitialized (arbitrary) data of the given shape, dtype, and order. Object arrays will be initialized to None.
Example: numpy.empty() function
>>> import numpy as np
>>> np.empty(2)
array([ 6.95033087e-310, 1.69970835e-316])
>>> np.empty(32)
array([ 6.95033087e-310, 1.65350412e-316, 6.95032869e-310,
6.95032869e-310, 6.95033051e-310, 6.95033014e-310,
6.95033165e-310, 6.95033167e-310, 6.95033163e-310,
6.95032955e-310, 6.95033162e-310, 6.95033166e-310,
6.95033160e-310, 6.95033163e-310, 6.95033162e-310,
6.95033167e-310, 6.95033167e-310, 6.95033167e-310,
6.95033167e-310, 6.95033158e-310, 6.95033160e-310,
6.95033164e-310, 6.95033162e-310, 6.95033051e-310,
6.95033161e-310, 6.95033051e-310, 6.95033013e-310,
6.95033166e-310, 6.95033161e-310, 2.97403466e+289,
7.55774284e+091, 1.31611495e+294])
>>> np.empty([2, 2])
array([[7.74860419e-304, 8.32155212e-317],
[0.00000000e+000, 0.00000000e+000]])
>>> np.empty([2, 3])
array([[ 6.95033087e-310, 1.68240973e-316, 6.95032825e-310],
[ 6.95032825e-310, 6.95032825e-310, 6.95032825e-310]])
Pictorial Presentation:

Example: numpy.empty() where data-type for the array is int
>>>import numpy as np
>>> np.empty([2, 2], dtype=int)
array([[140144669465496, 16250304],
[140144685653488, 140144685468840]])
>>> np.empty([2, 2], dtype=float)
array([[2.37015306e-316, 7.37071328e-315],
[9.06655519e-317, 2.00753892e-317]])
Pictorial Presentation:

Python - NumPy Code Editor:
Previous: NumPy array Home
Next: empty_like()
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework