MySQL LOWER() function
LOWER() function
MySQL LOWER() converts all the characters in a string to lowercase characters.
Syntax:
LOWER(str)
Argument:
Name | Description |
---|---|
str | A string whose characters are going to be converted to lowercase. |
Syntax Diagram:
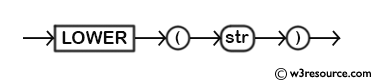
MySQL Version: 5.6
Video Presentation:
Example: MySQL LOWER() function
The following MySQL statement returns the given string after converting all of its characters in lowercase.
Code:
SELECT LOWER('MYTESTSTRING');
Sample Output:
mysql> SELECT LOWER('MYTESTSTRING'); +-----------------------+ | LOWER('MYTESTSTRING') | +-----------------------+ | myteststring | +-----------------------+ 1 row in set (0.01 sec)
Example of LOWER() on column of a table
The following MySQL statement returns those rows from the publisher table whose publishers does not belong to the USA. The column pub_name and after converting the pub_name column in lowercase is displayed in the output.
Code:
SELECT pub_name,LOWER(pub_name)
FROM publisher
WHERE country<>'USA';
Sample table : publisher
Sample Output:
mysql> SELECT pub_name,LOWER(pub_name) -> FROM publisher -> WHERE country<>'USA'; +------------------------------+------------------------------+ | pub_name | LOWER(pub_name) | +------------------------------+------------------------------+ | BPP Publication | bpp publication | | New Harrold Publication | new harrold publication | | Ultra Press Inc. | ultra press inc. | | Pieterson Grp. of Publishers | pieterson grp. of publishers | | Novel Publisher Ltd. | novel publisher ltd. | +------------------------------+------------------------------+ 5 rows in set (0.00 sec)
PHP script:
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>example lower function - php mysql examples | w3resource</title>
<meta name="description" content="example lower function - php mysql examples | w3resource">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-12">
<h2>List of publishers those who don't belong to USA. Right column shows the name of of the Publisher in lowercase:</h2><table class='table table-bordered'><tr>
<th>Publishers name</th><th>Publishers name in lowercase</th>
</tr>
<?php
$hostname="your_hostname";
$username="your_username";
$password="your_password";
$db = "your_dbname";
$dbh = new PDO("mysql:host=$hostname;dbname=$db", $username, $password);
foreach($dbh->query('SELECT pub_name,LOWER(pub_name)
FROM publisher
WHERE country<>"USA"') as $row) {
echo "<tr>";
echo "<td>" . $row['pub_name'] . "</td>";
echo "<td>" . $row['LOWER(pub_name)'] . "</td>";
echo "</tr>";
}
?></tbody></table>
</div>
</div>
</div>
</body>
</html>
JSP script:
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<%@ page import="java.sql.*" %>
<%@ page import="java.io.*" %>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>mysql-lower-function</title>
</head>
<body>
<%
try {
Class.forName("com.mysql.jdbc.Driver").newInstance();
String Host = "jdbc:mysql://localhost:3306/w3resour_bookinfo";
Connection connection = null;
Statement statement = null;
ResultSet rs = null;
connection = DriverManager.getConnection(Host, "root", "datasoft123");
statement = connection.createStatement();
String Data ="SELECT pub_name,LOWER(pub_name) FROM publisher WHERE country<>'USA'";
rs = statement.executeQuery(Data);
%>
<TABLE border="1">
<tr width="10" bgcolor="#9979">
<td>Publishers name</td>
<td>Publishers name in lowercase</td>
</tr>
<%
while (rs.next()) {
%>
<TR>
<TD><%=rs.getString("pub_name")%></TD>
<TD><%=rs.getString("LOWER(pub_name)")%></TD>
</TR>
<% } %>
</table>
<%
rs.close();
statement.close();
connection.close();
} catch (Exception ex) {
out.println("Cant connect to database.");
}
%>
</body>
</html>
Online Practice Editor:
All String Functions
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework