MySQL LIKE operator
LIKE operator
MySQL LIKE operator along with WILDCARDS finds a string of a specified pattern within another string.
In a more technical note, LIKE operator does pattern matching using simple regular expression comparison.
This is a table which describes the wildcards used with MySQL LIKE operator -
Wildcards | Description |
---|---|
% | Matches any number of characters including zero. |
_ | Matches exactly one character. |
Syntax:
LIKE pat
Argument
Name | Description |
---|---|
pat | A pattern which is to be matched. |
MySQL Version: 5.6
Video Presentation
Example of MySQL LIKE operator with wildcard (%) matching from the beginning
The following MySQL statement will return those rows from the table author in which the name of the author starts with the character ‘W’.
Code:
SELECT *
FROM author
WHERE aut_name LIKE 'W%';
Relational Algebra Expression:

Relational Algebra Tree:
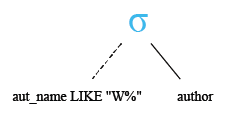
Sample table: author
Sample Output:
mysql> SELECT * -> FROM author -> WHERE aut_name LIKE 'W%'; +--------+-----------------+---------+-----------+ | aut_id | aut_name | country | home_city | +--------+-----------------+---------+-----------+ | AUT001 | William Norton | UK | Cambridge | | AUT002 | William Maugham | Canada | Toronto | | AUT003 | William Anthony | UK | Leeds | +--------+-----------------+---------+-----------+ 3 rows in set (0.04 sec)
PHP script
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>example php mysql like function | w3resource</title>
<meta name="description" content="example php mysql like function">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-12">
<h2>List of authors with all their detail available, where name of the author starts with 'W':</h2>
<table class='table table-bordered'>
<tr>
<th>Author's ID</th><th>Author's name</th><th>Country</th><th>Home City</th>
</tr>
<?php
$hostname="your_hostname";
$username="your_username";
$password="your_password";
$db = "your_dbname";
$dbh = new PDO("mysql:host=$hostname;dbname=$db", $username, $password);
foreach($dbh->query('SELECT * FROM author WHERE aut_name LIKE "W%"') as $row) {
echo "<tr>";
echo "<td>" . $row['aut_id'] . "</td>";
echo "<td>" . $row['aut_name'] . "</td>";
echo "<td>" . $row['country'] . "</td>";
echo "<td>" . $row['home_city'] . "</td>";
echo "</tr>";
}
?>
</tbody></table>
</div>
</div>
</div>
</body>
</html>
JSP script
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<%@ page import="java.sql.*" %>
<%@ page import="java.io.*" %>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>example-like-function</title>
</head>
<body>
<%
try {
Class.forName("com.mysql.jdbc.Driver").newInstance();
String Host = "jdbc:mysql://localhost:3306/w3resour_bookinfo";
Connection connection = null;
Statement statement = null;
ResultSet rs = null;
connection = DriverManager.getConnection(Host, "root", "datasoft123");
statement = connection.createStatement();
String Data ="SELECT * FROM author WHERE aut_name LIKE 'W%'";
rs = statement.executeQuery(Data);
%>
<TABLE border="1">
<tr width="10" bgcolor="#9979">
<td>Author's ID</td>
<td>Author's name</td>
<td>Country</td>
<td>Home City</td>
</tr>
<%
while (rs.next()) {
%>
<TR>
<TD><%=rs.getString("aut_id")%></TD>
<TD><%=rs.getString("aut_name")%></TD>
<TD><%=rs.getString("country")%></TD>
<TD><%=rs.getString("home_city")%></TD>
</TR>
<% } %>
</table>
<%
rs.close();
statement.close();
connection.close();
} catch (Exception ex) {
out.println("Cant connect to database.");
}
%>
</body>
</html>
Example of MySQL LIKE operator with wildcard (%) matching from the end
The following MySQL statement will return those rows from the table author in which the name of the author ends with the substring ‘on’.
Code:
SELECT * FROM author
WHERE aut_name LIKE '%on';
Relational Algebra Expression:

Relational Algebra Tree:
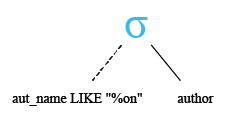
Sample table: author
Sample Output:
mysql> SELECT * -> FROM author -> WHERE aut_name LIKE '%on'; +--------+----------------+---------+-----------+ | aut_id | aut_name | country | home_city | +--------+----------------+---------+-----------+ | AUT001 | William Norton | UK | Cambridge | | AUT006 | Thomas Merton | USA | New York | | AUT007 | Piers Gibson | UK | London | | AUT010 | Joseph Milton | USA | Houston | +--------+----------------+---------+-----------+ 4 rows in set (0.00 sec)
PHP script
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Example of like function | w3resource</title>
<meta name="description" content="Example of like function">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-12">
<h2>List of authors with all their detail available, where name of the author ends with 'on':</h2>
<table class='table table-bordered'>
<tr>
<th>Author's ID</th><th>Author's name</th><th>Country</th><th>Home City</th>
</tr>
<?php
$hostname="your_hostname";
$username="your_username";
$password="your_password";
$db = "your_dbname";
$dbh = new PDO("mysql:host=$hostname;dbname=$db", $username, $password);
foreach($dbh->query('SELECT * FROM author WHERE aut_name LIKE "%on"') as $row) {
echo "<tr>";
echo "<td>" . $row['aut_id'] . "</td>";
echo "<td>" . $row['aut_name'] . "</td>";
echo "<td>" . $row['country'] . "</td>";
echo "<td>" . $row['home_city'] . "</td>";
echo "</tr>";
}
?>
</tbody></table>
</div>
</div>
</div>
</body>
</html>
Example of MySQL LIKE operator with wildcard (%) matching within the string
The following MySQL statement will return those rows from the table author in which the name of the author contains ‘k’.
Code:
SELECT * FROM author
WHERE aut_name LIKE '%k%';
Relational Algebra Expression:

Relational Algebra Tree:
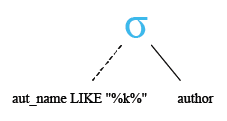
Sample table: author
Sample Output:
mysql> SELECT * -> FROM author -> WHERE aut_name LIKE '%k%'; +--------+---------------+---------+-----------+ | aut_id | aut_name | country | home_city | +--------+---------------+---------+-----------+ | AUT008 | Nikolai Dewey | USA | Atlanta | | AUT012 | Evan Hayek | Canada | Vancouver | +--------+---------------+---------+-----------+ 2 rows in set (0.00 sec)
Example of MySQL LIKE operator with wildcard (_) underscore
The following MySQL statement will return those rows from the table author in which the length of the author’s name is exactly 12 characters. Twelve ‘_’ have been used to indicate 12 characters.
Code:
SELECT * FROM author
WHERE aut_name LIKE '____________';
Relational Algebra Expression:

Relational Algebra Tree:
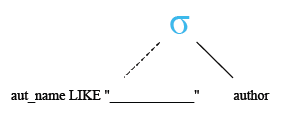
Sample table: author
Sample Output:
mysql> SELECT * -> FROM author -> WHERE aut_name LIKE '____________'; +--------+--------------+---------+-----------+ | aut_id | aut_name | country | home_city | +--------+--------------+---------+-----------+ | AUT007 | Piers Gibson | UK | London | | AUT015 | Butler Andre | USA | Florida | +--------+--------------+---------+-----------+ 2 rows in set (0.00 sec)
Online Practice Editor:
All String Functions
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework