Java Exercises: Find the distinct ways you can climb to the top
Java Basic: Exercise-134 with Solution
Write a Java program to find the distinct ways you can climb to the top (n steps to reach to the top) of stairs. Each time you can either climb 1 or 2 steps.
Example: n = 5 a) 1+1+1+1+1 = 5 b) 1+1+1+2 = 5 c) 1+2+2 = 5 d) 2+2+1 = 5 e) 2+1+1+1 = 5 f) 2+1+2 = 5 g) 1+2+1+1 = 5 h) 1+1+2+1 = 5
Pictorial Presentation:
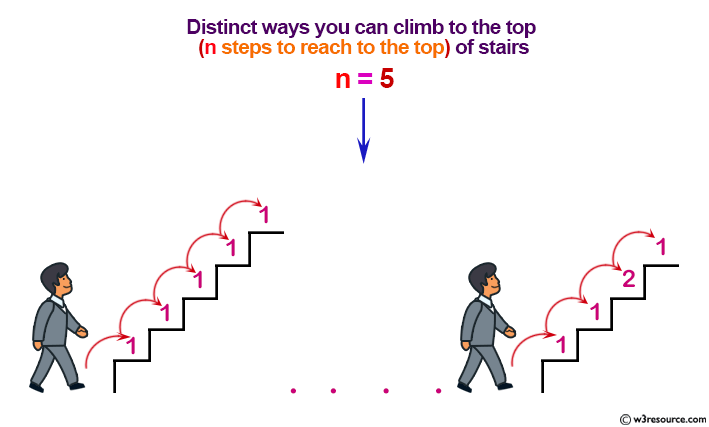
Sample Solution:
Java Code:
class Solution {
public static int climbStairs(int n) {
if (n <= 1) {
return 1;
}
int[] s_case = new int[n + 1];
s_case[0] = 1;
s_case[1] = 1;
for (int i = 2; i <= n; i++) {
s_case[i] = s_case[i - 1] + s_case[i - 2];
}
return s_case[n];
}
/* Driver program to test above functions */
public static void main(String[] args) {
int steps = 5;
System.out.println("Distinct ways can you climb to the top: "+climbStairs(steps));
}
}
Sample Output:
Distinct ways can you climb to the top: 8
Flowchart:
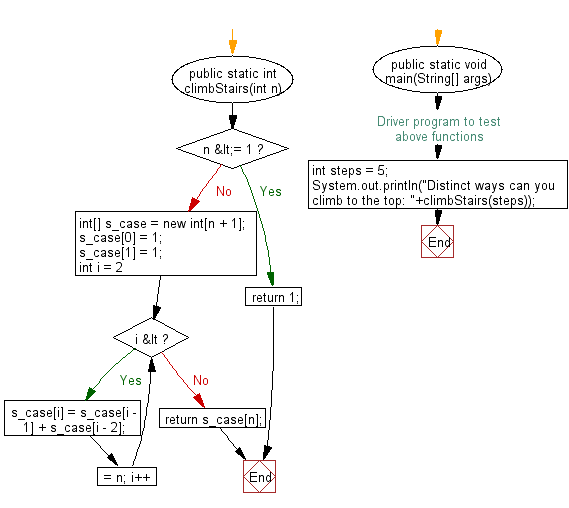
Java Code Editor:
Contribute your code and comments through Disqus.
Previous:Write a Java program to find a path from top left to bottom in right direction which minimizes the sum of all numbers along its path.
Next: Write a Java program to remove duplicates from a sorted linked list
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework