Java Exercises: Find a path from top left to bottom in right direction
Java Basic: Exercise-133 with Solution
Write a Java program to find a path from top left to bottom in right direction which minimizes the sum of all numbers along its path.
Note: Move either down or right at any point in time.
Pictorial Presentation:
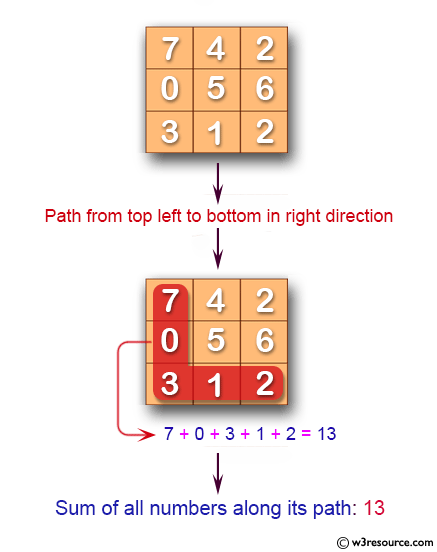
Sample Solution:
Java Code:
public class Solution {
public static int minPathSum(int[][] grid) {
if (grid == null || grid.length == 0 || grid[0] == null || grid[0].length == 0) {
return 0;
}
int m = grid.length;
int n = grid[0].length;
int[][] temp = new int[m][n];
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
if (i == 0 && j == 0) {
temp[i][j] = grid[i][j];
continue;
}
// Compute temp
int from_up = i == 0 ? Integer.MAX_VALUE : temp[i - 1][j];
int from_left = j == 0 ? Integer.MAX_VALUE : temp[i][j - 1];
temp[i][j] = Math.min(from_up, from_left) + grid[i][j];
}
}
return temp[m - 1][n - 1];
}
/* Driver program to test above functions */
public static void main(String[] args) {
int[][] grid = new int[][] {{7,4,2},
{0,5,6},
{3,1,2}};
System.out.println("Sum of all numbers along its path: "+minPathSum(grid));
}
}
Sample Output:
Sum of all numbers along its path: 13
Flowchart:

Java Code Editor:
Contribute your code and comments through Disqus.
Previous:Write a Java program to find the new length of a given sorted array where duplicate elements appeared at most twice.
Next: Write a Java program to find the distinct ways you can climb to the top (n steps to reach to the top) of stairs. Each time you can either climb 1 or 2 steps.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework