Java Exercises: Remove duplicates from a sorted linked list
Java Basic: Exercise-135 with Solution
Write a Java program to remove duplicates from a sorted linked list.
Pictorial Presentation:
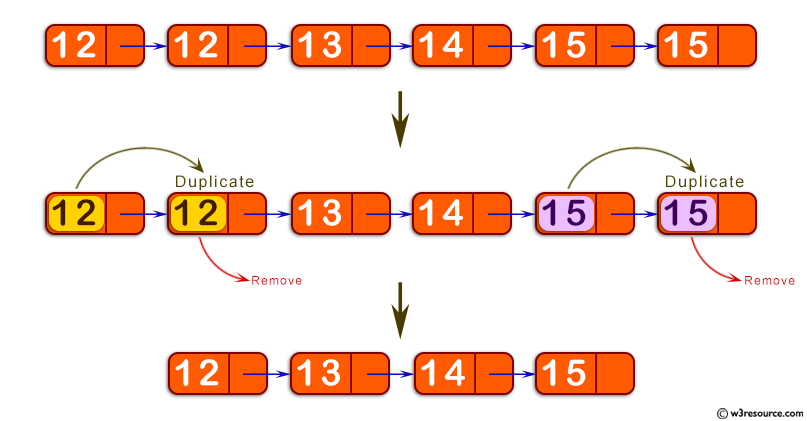
Sample Solution:
Java Code:
class LinkedList
{
Node head; // head of list
/* Linked list Node*/
class Node
{
int data;
Node next;
Node(int d) {data = d; next = null; }
}
void remove_Duplicates()
{
Node current = head;
Node next_next;
if (head == null)
return;
while (current.next != null) {
if (current.data == current.next.data) {
next_next = current.next.next;
current.next = null;
current.next = next_next;
}
else
current = current.next;
}
}
// In front of the list insert a new Node
public void push(int new_data)
{
Node new_node = new Node(new_data);
new_node.next = head;
head = new_node;
}
/* Function to print linked list */
void printList()
{
Node temp = head;
while (temp != null)
{
System.out.print(temp.data);
if (temp.next != null)
{
System.out.print("->");
}
temp = temp.next;
}
System.out.println();
}
/* Driver program to test above functions */
public static void main(String args[])
{
LinkedList l_list = new LinkedList();
// Insert data into LinkedList
l_list.push(17);
l_list.push(17);
l_list.push(16);
l_list.push(15);
l_list.push(15);
l_list.push(14);
l_list.push(13);
l_list.push(12);
l_list.push(12);
System.out.println("Original List with duplicate elements:");
l_list.printList();
l_list.remove_Duplicates();
System.out.println("After removing duplicates from the said list:");
l_list.printList();
}
}
Sample Output:
Original List with duplicate elements: 12->12->13->14->15->15->16->17->17 After removing duplicates from the said list: 12->13->14->15->16->17
Flowchart:
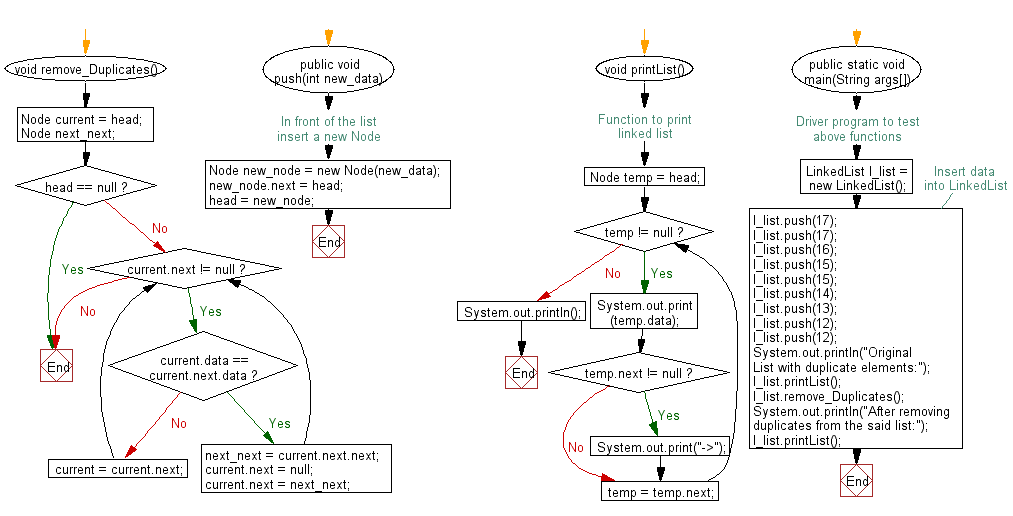
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to find the distinct ways you can climb to the top (n steps to reach to the top) of stairs. Each time you can either climb 1 or 2 steps.
Next: Write a Java program to find possible unique paths from top-left corner to bottom-right corner of a given grid (m x n).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework