C# Sharp Exercises: Compare three versions of the letter "I"
C# Sharp String: Exercise-29 with Solution
Write a C# Sharp program to compare three versions of the letter "I". The results are affected by the choice of culture, whether case is ignored, and whether an ordinal comparison is performed.
Sample Solution:-
C# Sharp Code:
// This example demonstrates the
// System.String.Compare(String, String, StringComparison) method.
using System;
using System.Threading;
class Example29
{
public static void Main()
{
string intro = "Compare three versions of the letter I using different " +
"values of StringComparison.";
// Define an array of strings where each element contains a version of the
// letter I. (An array of strings is used so you can easily modify this
// code example to test additional or different combinations of strings.)
string[] threeIs = new string[3];
// LATIN SMALL LETTER I (U+0069)
threeIs[0] = "\u0069";
// LATIN SMALL LETTER DOTLESS I (U+0131)
threeIs[1] = "\u0131";
// LATIN CAPITAL LETTER I (U+0049)
threeIs[2] = "\u0049";
string[] unicodeNames =
{
"LATIN SMALL LETTER I (U+0069)",
"LATIN SMALL LETTER DOTLESS I (U+0131)",
"LATIN CAPITAL LETTER I (U+0049)"
};
StringComparison[] scValues = {
StringComparison.CurrentCulture,
StringComparison.CurrentCultureIgnoreCase,
StringComparison.InvariantCulture,
StringComparison.InvariantCultureIgnoreCase,
StringComparison.Ordinal,
StringComparison.OrdinalIgnoreCase };
//
Console.Clear();
Console.WriteLine(intro);
// Display the current culture because the culture-specific comparisons
// can produce different results with different cultures.
Console.WriteLine("The current culture is {0}.\n",
Thread.CurrentThread.CurrentCulture.Name);
// Determine the relative sort order of three versions of the letter I.
foreach (StringComparison sc in scValues)
{
Console.WriteLine("StringComparison.{0}:", sc);
// LATIN SMALL LETTER I (U+0069) : LATIN SMALL LETTER DOTLESS I (U+0131)
Test(0, 1, sc, threeIs, unicodeNames);
// LATIN SMALL LETTER I (U+0069) : LATIN CAPITAL LETTER I (U+0049)
Test(0, 2, sc, threeIs, unicodeNames);
// LATIN SMALL LETTER DOTLESS I (U+0131) : LATIN CAPITAL LETTER I (U+0049)
Test(1, 2, sc, threeIs, unicodeNames);
Console.WriteLine();
}
}
protected static void Test(int x, int y,
StringComparison comparison,
string[] testI, string[] testNames)
{
string resultFmt = "{0} is {1} {2}";
string result = "equal to";
int cmpValue = 0;
//
cmpValue = String.Compare(testI[x], testI[y], comparison);
if (cmpValue < 0)
result = "less than";
else if (cmpValue > 0)
result = "greater than";
Console.WriteLine(resultFmt, testNames[x], result, testNames[y]);
}
}
Sample Output:
Compare three versions of the letter I using different values of StringComparison. The current culture is en-US. StringComparison.CurrentCulture: LATIN SMALL LETTER I (U+0069) is less than LATIN SMALL LETTER DOTLESS I (U+0131) LATIN SMALL LETTER I (U+0069) is less than LATIN CAPITAL LETTER I (U+0049) LATIN SMALL LETTER DOTLESS I (U+0131) is greater than LATIN CAPITAL LETTER I (U+0049) StringComparison.CurrentCultureIgnoreCase: LATIN SMALL LETTER I (U+0069) is less than LATIN SMALL LETTER DOTLESS I (U+0131) LATIN SMALL LETTER I (U+0069) is equal to LATIN CAPITAL LETTER I (U+0049) LATIN SMALL LETTER DOTLESS I (U+0131) is greater than LATIN CAPITAL LETTER I (U+0049) StringComparison.InvariantCulture: LATIN SMALL LETTER I (U+0069) is less than LATIN SMALL LETTER DOTLESS I (U+0131) LATIN SMALL LETTER I (U+0069) is less than LATIN CAPITAL LETTER I (U+0049) LATIN SMALL LETTER DOTLESS I (U+0131) is greater than LATIN CAPITAL LETTER I (U+0049) StringComparison.InvariantCultureIgnoreCase: LATIN SMALL LETTER I (U+0069) is less than LATIN SMALL LETTER DOTLESS I (U+0131) LATIN SMALL LETTER I (U+0069) is equal to LATIN CAPITAL LETTER I (U+0049) LATIN SMALL LETTER DOTLESS I (U+0131) is greater than LATIN CAPITAL LETTER I (U+0049) StringComparison.Ordinal: LATIN SMALL LETTER I (U+0069) is less than LATIN SMALL LETTER DOTLESS I (U+0131) LATIN SMALL LETTER I (U+0069) is greater than LATIN CAPITAL LETTER I (U+0049) LATIN SMALL LETTER DOTLESS I (U+0131) is greater than LATIN CAPITAL LETTER I (U+0049) StringComparison.OrdinalIgnoreCase: LATIN SMALL LETTER I (U+0069) is equal to LATIN SMALL LETTER DOTLESS I (U+0131) LATIN SMALL LETTER I (U+0069) is equal to LATIN CAPITAL LETTER I (U+0049) LATIN SMALL LETTER DOTLESS I (U+0131) is equal to LATIN CAPITAL LETTER I (U+0049)
Flowchart :
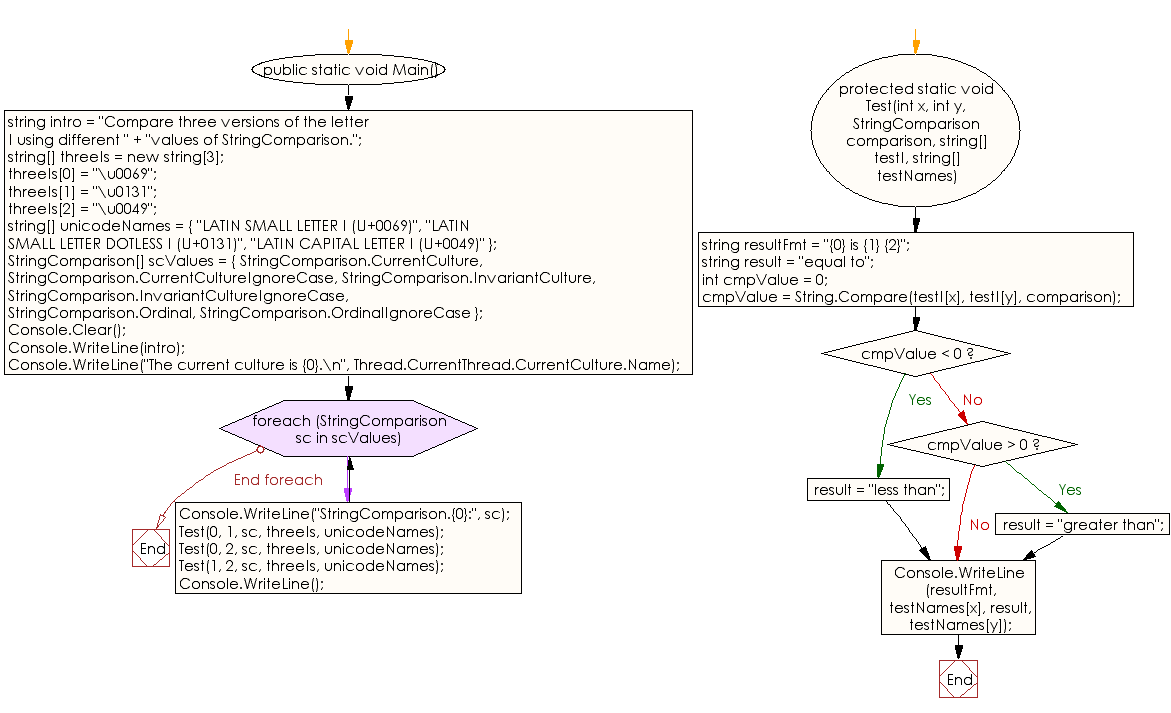
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compare two strings in following three different ways produce three different results.
Next: Write a C# Sharp program to demonstrate that CompareOrdinal and Compare use different sort orders.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework