C# Sharp Exercises: Compare two strings in different ways produce three different results
C# Sharp String: Exercise-28 with Solution
Write a C# Sharp program to compare two strings in following three different ways produce three different results.
a. using linguistic comparison for the en-US culture.
b. using linguistic case-sensitive comparison for the en-US culture.
c. using an ordinal comparison. It illustrates how the three methods of comparison.
Sample Solution:-
C# Sharp Code:
using System;
using System.Globalization;
public class Example28
{
public static void Main()
{
string str1 = "sister";
string str2 = "Sister";
string relation;
int result;
// Cultural (linguistic) comparison.
result = String.Compare(str1, str2, new CultureInfo("en-US"),
CompareOptions.None);
if (result > 0)
relation = "comes after";
else if (result == 0)
relation = "is the same as";
else
relation = "comes before";
Console.WriteLine("'{0}' {1} '{2}'.",
str1, relation, str2);
// Cultural (linguistic) case-insensitive comparison.
result = String.Compare(str1, str2, new CultureInfo("en-US"),
CompareOptions.IgnoreCase);
if (result > 0)
relation = "comes after";
else if (result == 0)
relation = "is the same as";
else
relation = "comes before";
Console.WriteLine("'{0}' {1} '{2}'.",
str1, relation, str2);
// Culture-insensitive ordinal comparison.
result = String.CompareOrdinal(str1, str2);
if (result > 0)
relation = "comes after";
else if (result == 0)
relation = "is the same as";
else
relation = "comes before";
Console.WriteLine("'{0}' {1} '{2}'.",
str1, relation, str2);
}
}
Sample Output:
'sister' comes before 'Sister'. 'sister' is the same as 'Sister'. 'sister' comes after 'Sister'.
Flowchart:
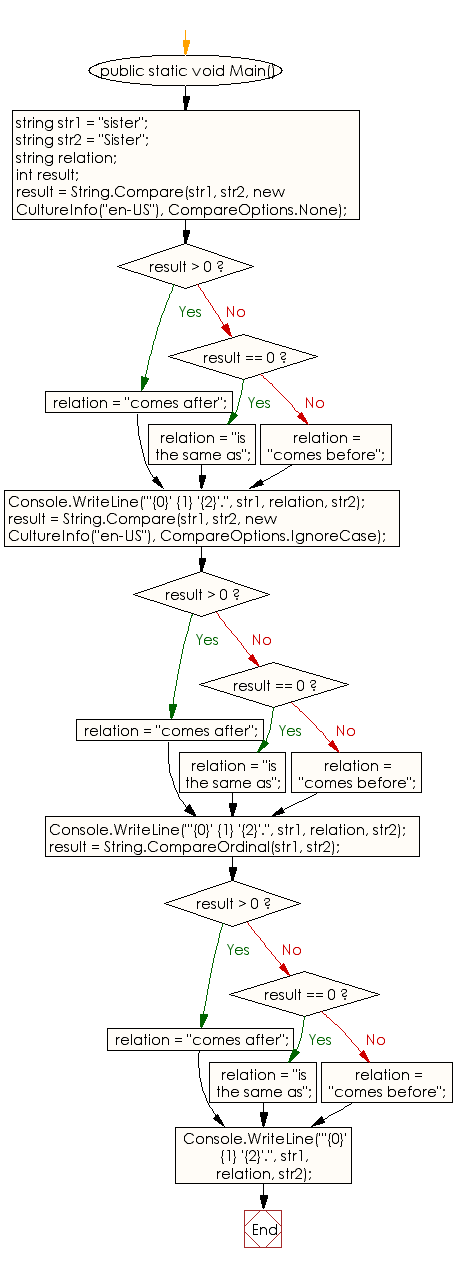
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to demonstrate how culture can affect a comparison.
Next: Write a C# Sharp program to compare three versions of the letter "I". The results are affected by the choice of culture, whether case is ignored, and whether an ordinal comparison is performed.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework