C# Sharp Exercises: Demonstrate that compare ordinal and compare use different sort orders
C# Sharp String: Exercise-30 with Solution
Write a C# Sharp program to demonstrate that compare ordinal and compare use different sort orders.
Sample Solution:-
C# Sharp Code:
using System;
using System.Globalization;
class Example30
{
public static void Main(String[] args)
{
String strLow = "xyz";
String strCap = "XYZ";
String result = "equal to ";
int x = 0;
int pos = 1;
// The Unicode codepoint for 'b' is greater than the codepoint for 'B'.
x = String.CompareOrdinal(strLow, pos, strCap, pos, 1);
if (x < 0) result = "less than";
if (x > 0) result = "greater than";
Console.WriteLine("CompareOrdinal(\"{0}\"[{2}], \"{1}\"[{2}]):", strLow, strCap, pos);
Console.WriteLine(" '{0}' is {1} '{2}'", strLow[pos], result, strCap[pos]);
// In U.S. English culture, 'b' is linguistically less than 'B'.
x = String.Compare(strLow, pos, strCap, pos, 1, false, new CultureInfo("en-US"));
if (x < 0) result = "less than";
else if (x > 0) result = "greater than";
Console.WriteLine("Compare(\"{0}\"[{2}], \"{1}\"[{2}]):", strLow, strCap, pos);
Console.WriteLine(" '{0}' is {1} '{2}'", strLow[pos], result, strCap[pos]);
}
}
Sample Output:
CompareOrdinal("xyz"[1], "XYZ"[1]): 'y' is greater than 'Y' Compare("xyz"[1], "XYZ"[1]): 'y' is less than 'Y'
Flowchart :
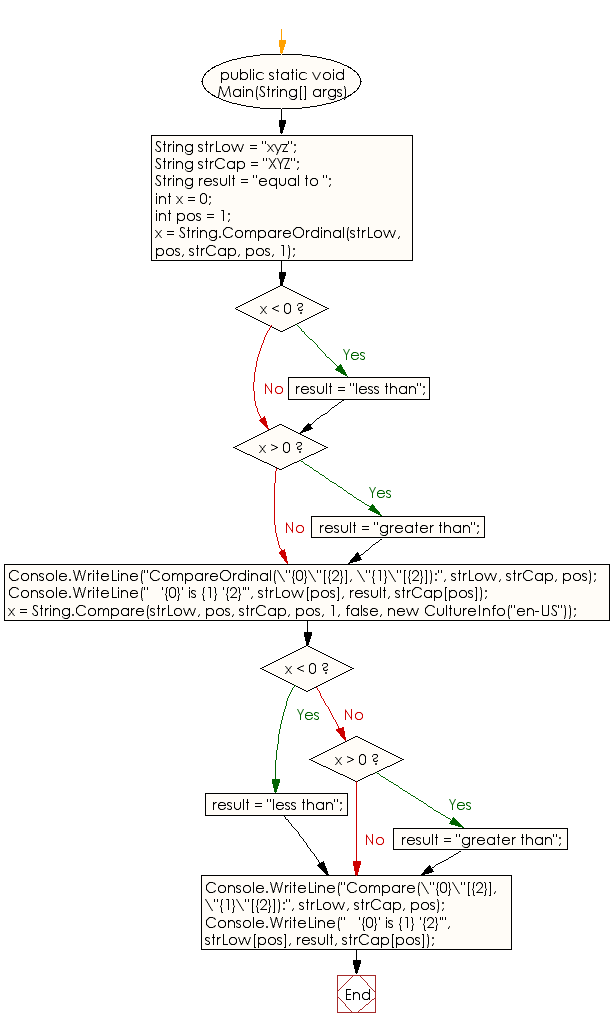
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to compare three versions of the letter "I". The results are affected by the choice of culture, whether case is ignored, and whether an ordinal comparison is performed.
Next: Write a C# Sharp program to perform and ordinal comparison of two strings that only differ in case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework