SQLite Exercise: Get the employee ID, names, salary in ascending order of salary
Write a query to get the employee ID, names (first_name, last_name), salary in ascending order of salary.
Sample table : employees
SQLite Code:
SELECT employee_id, first_name, last_name, salary
FROM employees ORDER BY salary;
Output:
employee_id first_name last_name salary ----------- ---------- ---------- ---------- 132 TJ Olson 2100 128 Steven Markle 2200 136 Hazel Philtanker 2200 127 James Landry 2400 135 Ki Gee 2400 119 Karen Colmenares 2500 131 James Marlow 2500 140 Joshua Patel 2500 144 Peter Vargas 2500 182 Martha Sullivan 2500 191 Randall Perkins 2500 118 Guy Himuro 2600 143 Randall Matos 2600 198 Donald OConnell 2600 199 Douglas Grant 2600 126 Irene Mikkilinen 2700 139 John Seo 2700 117 Sigal Tobias 2800 130 Mozhe Atkinson 2800 183 Girard Geoni 2800 195 Vance Jones 2800 116 Shelli Baida 2900 134 Michael Rogers 2900 190 Timothy Gates 2900 187 Anthony Cabrio 3000 197 Kevin Feeney 3000 115 Alexander Khoo 3100 142 Curtis Davies 3100 181 Jean Fleaur 3100 196 Alana Walsh 3100 125 Julia Nayer 3200 138 Stephen Stiles 3200 180 Winston Taylor 3200 194 Samuel McCain 3200 129 Laura Bissot 3300 133 Jason Mallin 3300 186 Julia Dellinger 3400 141 Trenna Rajs 3500 137 Renske Ladwig 3600 189 Jennifer Dilly 3600 188 Kelly Chung 3800 193 Britney Everett 3900 192 Sarah Bell 4000 185 Alexis Bull 4100 107 Diana Lorentz 4200 184 Nandita Sarchand 4200 200 Jennifer Whalen 4400 105 David Austin 4800 106 Valli Pataballa 4800 124 Kevin Mourgos 5800 104 Bruce Ernst 6000 202 Pat Fay 6000 173 Sundita Kumar 6100 167 Amit Banda 6200 179 Charles Johnson 6200 166 Sundar Ande 6400 123 Shanta Vollman 6500 203 Susan Mavris 6500 165 David Lee 6800 113 Luis Popp 6900 155 Oliver Tuvault 7000 161 Sarath Sewall 7000 178 Kimberely Grant 7000 164 Mattea Marvins 7200 172 Elizabeth Bates 7300 171 William Smith 7400 154 Nanette Cambrault 7500 160 Louise Doran 7500 111 Ismael Sciarra 7700 112 Jose Manue Urman 7800 122 Payam Kaufling 7900 120 Matthew Weiss 8000 153 Christophe Olsen 8000 159 Lindsey Smith 8000 110 John Chen 8200 121 Adam Fripp 8200 206 William Gietz 8300 177 Jack Livingston 8400 176 Jonathon Taylor 8600 175 Alyssa Hutton 8800 103 Alexander Hunold 9000 109 Daniel Faviet 9000 152 Peter Hall 9000 158 Allan McEwen 9000 151 David Bernstein 9500 157 Patrick Sully 9500 163 Danielle Greene 9500 170 Tayler Fox 9600 150 Peter Tucker 10000 156 Janette King 10000 169 Harrison Bloom 10000 204 Hermann Baer 10000 149 Eleni Zlotkey 10500 162 Clara Vishney 10500 114 Den Raphaely 11000 148 Gerald Cambrault 11000 174 Ellen Abel 11000 168 Lisa Ozer 11500 108 Nancy Greenberg 12000 147 Alberto Errazuriz 12000 205 Shelley Higgins 12000 201 Michael Hartstein 13000 146 Karen Partners 13500 145 John Russell 14000 101 Neena Kochhar 17000 102 Lex De Haan 17000 100 Steven King 24000
Relational Algebra Expression:

Relational Algebra Tree:
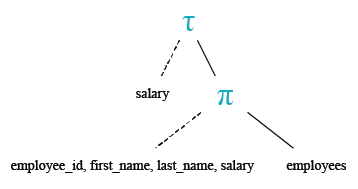
Practice SQLite Online
Model Database
Structure of 'hr' database :
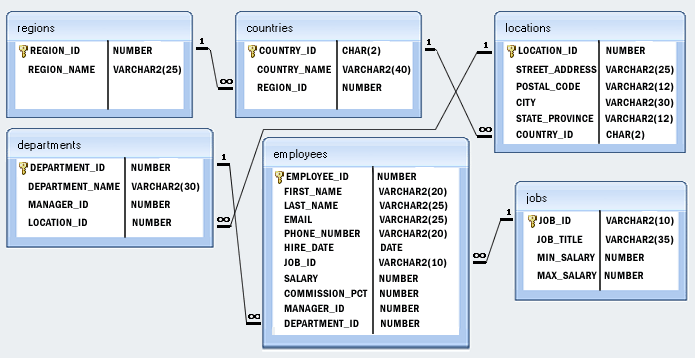
Improve this sample solution and post your code through Disqus.
Previous: Write a query to get the names (first_name, last_name), salary, PF of all the employees (PF is calculated as 12% of salary).
Next: Write a query to get the total salaries payable to employees.
What is the difficulty level of this exercise?
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework