SQLite Exercise: Get the names, salary, PF of all the employees
Write a query to get the names (first_name, last_name), salary, PF of all the employees (PF is calculated as 12% of salary).
Sample table : employees
SQLite Code :
SELECT first_name, last_name, salary, salary*.15 PF
FROM employees;
Output:
first_name last_name salary PF ---------- ---------- ---------- ---------- Steven King 24000 3600.0 Neena Kochhar 17000 2550.0 Lex De Haan 17000 2550.0 Alexander Hunold 9000 1350.0 Bruce Ernst 6000 900.0 David Austin 4800 720.0 Valli Pataballa 4800 720.0 Diana Lorentz 4200 630.0 Nancy Greenberg 12000 1800.0 Daniel Faviet 9000 1350.0 John Chen 8200 1230.0 Ismael Sciarra 7700 1155.0 Jose Manue Urman 7800 1170.0 Luis Popp 6900 1035.0 Den Raphaely 11000 1650.0 Alexander Khoo 3100 465.0 Shelli Baida 2900 435.0 Sigal Tobias 2800 420.0 Guy Himuro 2600 390.0 Karen Colmenares 2500 375.0 Matthew Weiss 8000 1200.0 Adam Fripp 8200 1230.0 Payam Kaufling 7900 1185.0 Shanta Vollman 6500 975.0 Kevin Mourgos 5800 870.0 Julia Nayer 3200 480.0 Irene Mikkilinen 2700 405.0 James Landry 2400 360.0 Steven Markle 2200 330.0 Laura Bissot 3300 495.0 Mozhe Atkinson 2800 420.0 James Marlow 2500 375.0 TJ Olson 2100 315.0 Jason Mallin 3300 495.0 Michael Rogers 2900 435.0 Ki Gee 2400 360.0 Hazel Philtanker 2200 330.0 Renske Ladwig 3600 540.0 Stephen Stiles 3200 480.0 John Seo 2700 405.0 Joshua Patel 2500 375.0 Trenna Rajs 3500 525.0 Curtis Davies 3100 465.0 Randall Matos 2600 390.0 Peter Vargas 2500 375.0 John Russell 14000 2100.0 Karen Partners 13500 2025.0 Alberto Errazuriz 12000 1800.0 Gerald Cambrault 11000 1650.0 Eleni Zlotkey 10500 1575.0 Peter Tucker 10000 1500.0 David Bernstein 9500 1425.0 Peter Hall 9000 1350.0 Christophe Olsen 8000 1200.0 Nanette Cambrault 7500 1125.0 Oliver Tuvault 7000 1050.0 Janette King 10000 1500.0 Patrick Sully 9500 1425.0 Allan McEwen 9000 1350.0 Lindsey Smith 8000 1200.0 Louise Doran 7500 1125.0 Sarath Sewall 7000 1050.0 Clara Vishney 10500 1575.0 Danielle Greene 9500 1425.0 Mattea Marvins 7200 1080.0 David Lee 6800 1020.0 Sundar Ande 6400 960.0 Amit Banda 6200 930.0 Lisa Ozer 11500 1725.0 Harrison Bloom 10000 1500.0 Tayler Fox 9600 1440.0 William Smith 7400 1110.0 Elizabeth Bates 7300 1095.0 Sundita Kumar 6100 915.0 Ellen Abel 11000 1650.0 Alyssa Hutton 8800 1320.0 Jonathon Taylor 8600 1290.0 Jack Livingston 8400 1260.0 Kimberely Grant 7000 1050.0 Charles Johnson 6200 930.0 Winston Taylor 3200 480.0 Jean Fleaur 3100 465.0 Martha Sullivan 2500 375.0 Girard Geoni 2800 420.0 Nandita Sarchand 4200 630.0 Alexis Bull 4100 615.0 Julia Dellinger 3400 510.0 Anthony Cabrio 3000 450.0 Kelly Chung 3800 570.0 Jennifer Dilly 3600 540.0 Timothy Gates 2900 435.0 Randall Perkins 2500 375.0 Sarah Bell 4000 600.0 Britney Everett 3900 585.0 Samuel McCain 3200 480.0 Vance Jones 2800 420.0 Alana Walsh 3100 465.0 Kevin Feeney 3000 450.0 Donald OConnell 2600 390.0 Douglas Grant 2600 390.0 Jennifer Whalen 4400 660.0 Michael Hartstein 13000 1950.0 Pat Fay 6000 900.0 Susan Mavris 6500 975.0 Hermann Baer 10000 1500.0 Shelley Higgins 12000 1800.0 William Gietz 8300 1245.0
Practice SQLite Online
Model Database
Structure of 'hr' database :
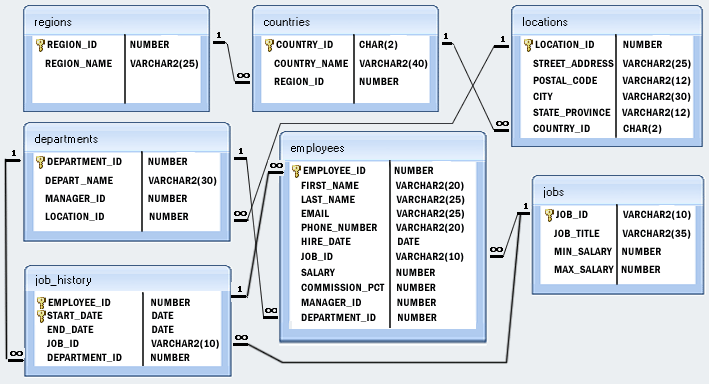
Improve this sample solution and post your code through Disqus.
Previous: Write a query to get all employee details from the employee table order by first name, descending.
Next: Write a query to get the employee ID, names (first_name, last_name), salary in ascending order of salary.