Python: Separate Parentheses Groups Perfectly
Python Programming Puzzles: Exercise-10 with Solution
Given a string consisting of whitespace and groups of matched parentheses, write a Python program to split it into groups of perfectly matched parentheses without any whitespace.
Input: ( ()) ((()()())) (()) () Output: ['(())', '((()()()))', '(())', '()'] Input: () (( ( )() ( )) ) ( ()) Output: ['()', '((()()()))', '(())']
Pictorial Presentation:
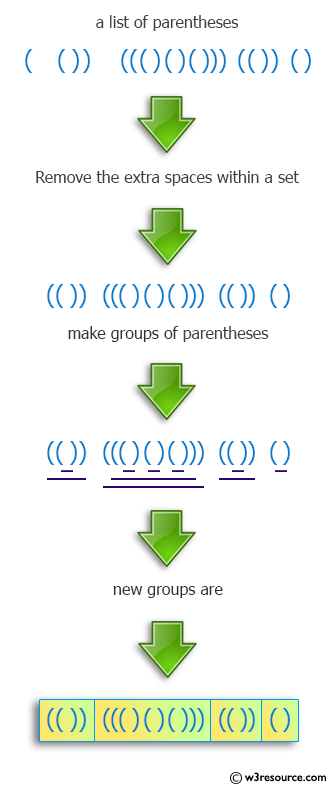
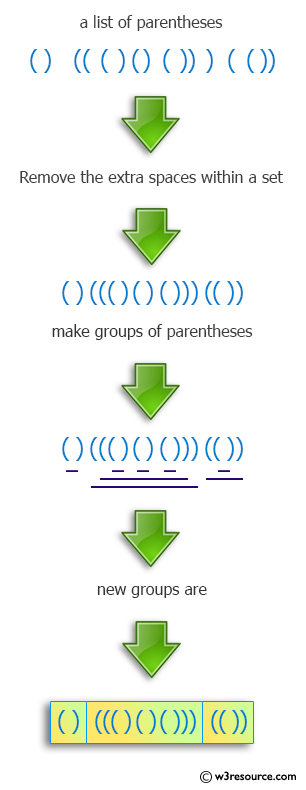
Sample Solution:
Python Code:
#License: https://bit.ly/3oLErEI
def test(combined):
ls = []
s2 = ""
for s in combined.replace(' ', ''):
s2 += s
if s2.count("(") == s2.count(")"):
ls.append(s2)
s2 = ""
return ls
combined = '( ()) ((()()())) (()) ()'
print("Parentheses string:")
print(combined)
print("Separate parentheses groups of the said string:")
print(test(combined))
combined = '() (( ( )() ( )) ) ( ())'
print("\nParentheses string:")
print(combined)
print("Separate parentheses groups of the said string:")
print(test(combined))
Sample Output:
Parentheses string: ( ()) ((()()())) (()) () Separate parentheses groups of the said string: ['(())', '((()()()))', '(())', '()'] Parentheses string: () (( ( )() ( )) ) ( ()) Separate parentheses groups of the said string: ['()', '((()()()))', '(())']
Flowchart:
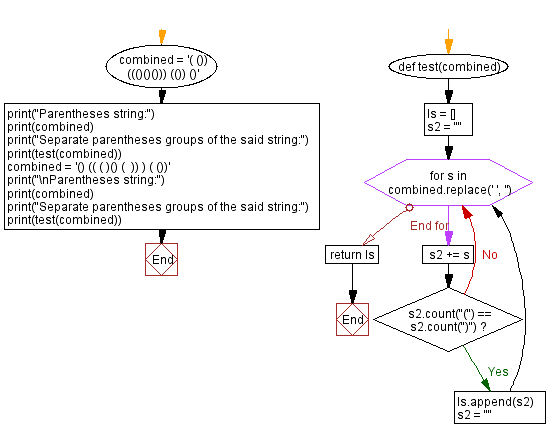
Visualize Python code execution:
The following tool visualize what the computer is doing step-by-step as it executes the said program:
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: List integers containing exactly three distinct values, such that no integer repeats twice consecutively.
Next: Find the indexes of numbers, below a given threshold.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Python: Tips of the Day
Find current directory and file's directory:
To get the full path to the directory a Python file is contained in, write this in that file:
import os dir_path = os.path.dirname(os.path.realpath(__file__))
(Note that the incantation above won't work if you've already used os.chdir() to change your current working directory, since the value of the __file__ constant is relative to the current working directory and is not changed by an os.chdir() call.)
To get the current working directory use
import os cwd = os.getcwd()
Documentation references for the modules, constants and functions used above:
- The os and os.path modules.
- The __file__ constant
- os.path.realpath(path) (returns "the canonical path of the specified filename, eliminating any symbolic links encountered in the path")
- os.path.dirname(path) (returns "the directory name of pathname path")
- os.getcwd() (returns "a string representing the current working directory")
- os.chdir(path) ("change the current working directory to path")
Ref: https://bit.ly/3fy0R6m
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework