JavaScript: Find the area of a triangle where lengths of the three of its sides are 5, 6, 7
JavaScript Basic: Exercise-4 with Solution
Write a JavaScript function to find the area of a triangle where lengths of the three of its sides are 5, 6, 7.
Sample Solution:
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset=utf-8 />
<title>The area of a triangle</title>
</head>
<body>
</body>
</html>
JavaScript Code:
var side1 = 5;
var side2 = 6;
var side3 = 7;
var s = (side1 + side2 + side3)/2;
var area = Math.sqrt(s*((s-side1)*(s-side2)*(s-side3)));
console.log(area);
Sample Output:
14.696938456699069
Explanation:
Calculate the area of a triangle of three given sides :
In geometry, Heron's formula named after Hero of Alexandria, gives the area of a triangle by requiring no arbitrary choice of side as base or vertex as origin, contrary to other formulas for the area of a triangle, such as half the base times the height or half the norm of a cross product of two sides.
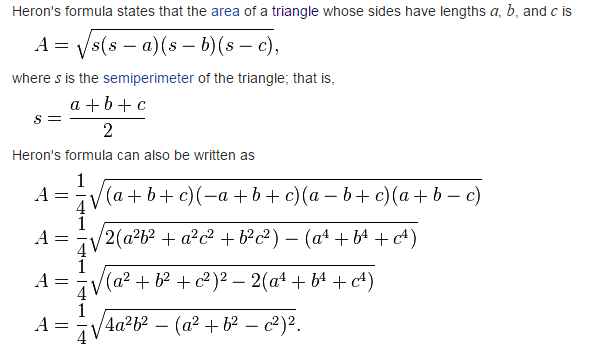
The Math.sqrt() function is used to get the square root of a number. If the value of the number is negative, Math.sqrt() returns NaN.
ES6 Version:
const side1 = 5;
const side2 = 6;
const side3 = 7;
const perimeter = (side1 + side2 + side3)/2;
const area = Math.sqrt(perimeter*((perimeter-side1)*(perimeter-side2)*(perimeter-side3)));
console.log(area);
Live Demo:
See the Pen JavaScript: Area of Triangle - basic-ex-4 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to get the current date.
Next: Rotate the string 'w3resource' in right direction by periodically removing one letter from the end of the string and attaching it to the front.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
JavaScript: Tips of the Day
How to insert an item into an array at a specific index (JavaScript)?
What you want is the splice function on the native array object.
arr.splice(index, 0, item); will insert item into arr at the specified index (deleting 0 items first, that is, it's just an insert). In this example we will create an array and add an element to it into index 2:
var arr = []; arr[0] = "Jani"; arr[1] = "Hege"; arr[2] = "Stale"; arr[3] = "Kai Jim"; arr[4] = "Borge"; console.log(arr.join()); arr.splice(2, 0, "Lene"); console.log(arr.join());
Ref: https://bit.ly/2BXbp04
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework