Java String Exercises: Print all permutations of a specified string with repetition
Java String: Exercise-35 with Solution
Write a Java program to print all permutations of a given string with repetition.
Pictorial Presentation:
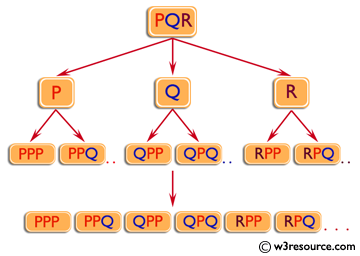
Sample Solution:
Java Code:
import java.util.*;
public class Main {
public static void main(String[] args) {
permutationWithRepeation("PQR");
}
private static void permutationWithRepeation(String str1) {
System.out.println("The given string is: PQR");
System.out.println("The permuted strings are:");
showPermutation(str1, "");
}
private static void showPermutation(String str1, String NewStringToPrint) {
if (NewStringToPrint.length() == str1.length()) {
System.out.println(NewStringToPrint);
return;
}
for (int i = 0; i < str1.length(); i++) {
showPermutation(str1, NewStringToPrint + str1.charAt(i));
}
}
}
Sample Output:
The given string is: PQR The permuted strings are: PPP PPQ PPR PQP PQQ PQR PRP PRQ PRR QPP QPQ QPR QQP QQQ QQR QRP QRQ QRR RPP RPQ RPR RQP RQQ RQR RRP RRQ RRR
Flowchart:
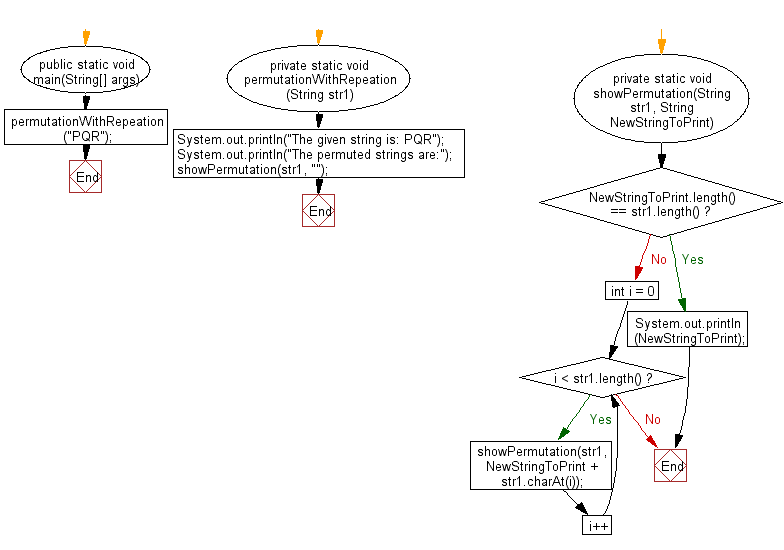
Visualize Java code execution (Python Tutor):
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to find the second most frequent character in a given string.
Next: Write a Java program to check whether two strings are interliving of a given string. Assuming that the unique characters in both strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework