Java Exercises: Check if a point (x, y) is in a triangle or not
Java Basic: Exercise-219 with Solution
Write a Java program to check if a point (x, y) is in a triangle or not. There is a triangle formed by three points.
Input:
x1,y1,x2,y2,x3,y3,xp,yp separated by a single space.
Sample Solution:
Java Code:
import java.util.*;
public class Main {
private double outer_product(double x, double y, double x1, double y1, double x2, double y2){
x1 = x1 - x;
y1 = y1 - y;
x2 = x2 - x;
y2 = y2 - y;
double s = x1 * y2 - y1 * x2;
return s;
}
public void point(){
Scanner sc = new Scanner(System.in);
double [] x = new double[3];
double [] y = new double[3];
System.out.println("Input (x1, y1)");
x[0] = sc.nextDouble();
y[0] = sc.nextDouble();
System.out.println("Input (x2, y2)");
x[1] = sc.nextDouble();
y[1] = sc.nextDouble();
System.out.println("Input (x3, y3)");
x[2] = sc.nextDouble();
y[2] = sc.nextDouble();
System.out.println("Input (xp, yp)");
double xp = sc.nextDouble();
double yp = sc.nextDouble();
boolean [] ans = new boolean[3];
for(int i=0; i < 3; i++){
ans[i] = outer_product(xp, yp, x[i], y[i], x[(i+1)%3], y[(i+1)%3]) > 0.0;
}
if(ans[0] == ans[1] && ans[1] == ans[2]){
}
else{
System.out.println("The point is outside the triangle.");
}
}
public static void main(String[] args) {
Main obj = new Main();
obj.point();
}
}
Sample Output:
Input (x1, y1) 2 6 Input (x2, y2) 3 5 Input (x3, y3) 4 6 Input (xp, yp) 5 6 The point is outside the triangle.
Flowchart:
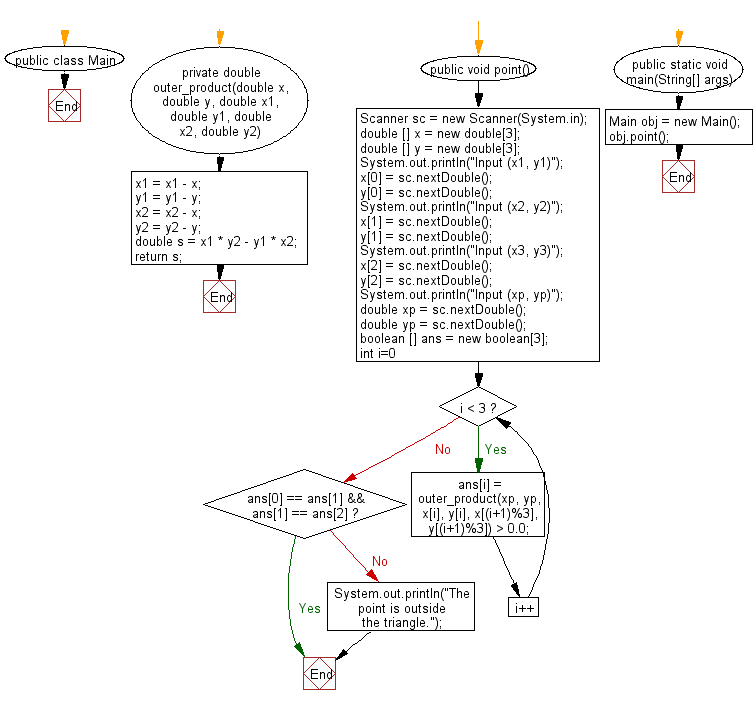
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to print the number of prime numbers which are less than or equal to a given integer.
Next: Write a Java program to compute and print sum of two given integers (more than or equal to zero). If given integers or the sum have more than 80 digits, print "overflow".
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework