Java Exercises: Compute the radius and the central coordinate of a circle
Java Basic: Exercise-218 with Solution
Write a Java program to compute the radius and the central coordinate (x, y) of a circle which is constructed by three given points on the plane surface.
Input:
x1,y1,x2,y2,x3,y3,xp,yp separated by a single space.
Sample Solution:
Java Code:
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.BufferedReader;
class Main {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.println("Input x1, y1, x2, y2, x3, y3 separated by a single space:");
String[] input = br.readLine().split(" ");
double x1 = Double.parseDouble(input[0]);
double y1 = Double.parseDouble(input[1]);
double x2 = Double.parseDouble(input[2]);
double y2 = Double.parseDouble(input[3]);
double x3 = Double.parseDouble(input[4]);
double y3 = Double.parseDouble(input[5]);
double A = x2 - x1;
double B = y2 - y1;
double p = (y2 * y2 - y1 * y1 + x2 * x2 - x1 * x1) / 2;
double C = x3 - x1;
double D = y3 - y1;
double q = (y3 * y3 - y1 * y1 + x3 * x3 - x1 * x1) / 2;
double X = (D * p - B * q) / (A * D - B * C);
double Y = (A * q - C * p) / (A * D - B * C);
double r = Math.sqrt(Math.pow(X - x1, 2.0) + Math.pow(Y - y1, 2.0));
System.out.println("\nRadius and the central coordinate:");
System.out.printf("%.3f (%.3f %.3f)", r, X, Y);
}
}
Sample Output:
Input x1, y1, x2, y2, x3, y3 separated by a single space: 5 6 4 8 7 9 Radius and the central coordinate: 1.821 (5.786 7.643)
Flowchart:
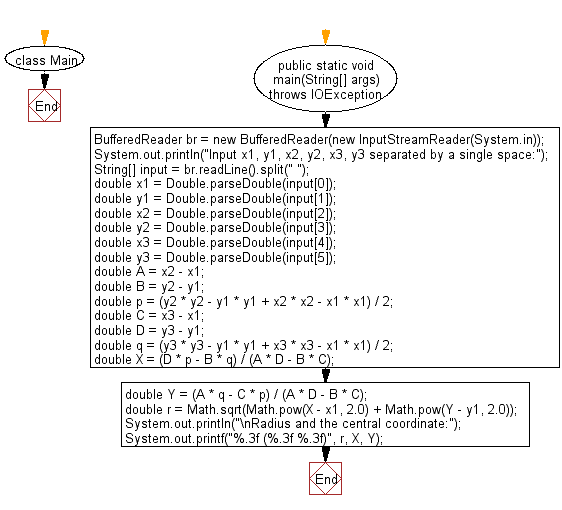
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to print the number of prime numbers which are less than or equal to a given integer.
Next: Write a Java program to check if a point (x, y) is in a triangle or not. There is a triangle formed by three points.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework