Java Exercises: Plus one to the number of a specified positive numbers represented as an array of digits
Java Basic: Exercise-179 with Solution
Write a Java program to plus one to the number of a given positive numbers represented as an array of digits.
Sample array: [9, 9, 9, 9] which represents 9999
Output: [1, 0, 0, 0, 0].
Sample Solution:
Java Code:
import java.util.*;
public class Solution {
public static void main(String[] args) {
int[] nums = {9,9,9,9};
System.out.println("Original array: " + Arrays.toString(nums));
System.out.println("Array of digits: " + Arrays.toString(plus_One_digit(nums)));
}
public static int[] plus_One_digit(int[] digits_nums) {
for (int i = digits_nums.length - 1; i > -1; --i) {
if (digits_nums[i] != 9) {
digits_nums[i] += 1;
for (int j = i + 1; j < digits_nums.length; ++j) {
digits_nums[j] = 0;
}
return digits_nums;
}
}
int[] result = new int[digits_nums.length + 1];
result[0] = 1;
return result;
}
}
Sample Output:
Original array: [9, 9, 9, 9] Array of digits: [1, 0, 0, 0, 0]
Flowchart:
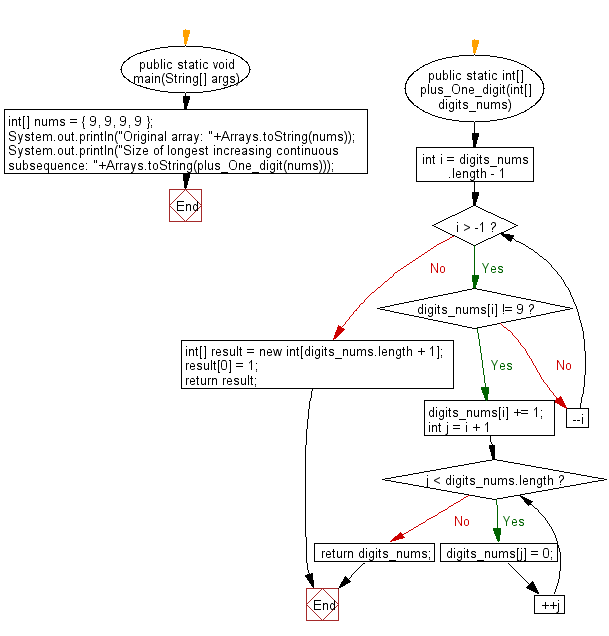
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to get a new binary tree with same structure and same value of a given binary tree.
Next: Write a Java program to swap every two adjacent nodes of a given linked list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework