C# Sharp Exercises: Check if a given string is a palindrome or not
C# Sharp Basic: Exercise-56 with Solution
Write a C# program to check if a given string is a palindrome or not.
Sample Example:
For 'aba' the output should be true
For 'abcd' the output should be false
Sample Solution:
C# Sharp Code:
using System;
public class Example
{
public static bool checkPalindrome(string inputString)
{
char[] c = inputString.ToCharArray();
Array.Reverse(c);
return new string(c).Equals(inputString);
}
public static void Main()
{
Console.WriteLine(checkPalindrome("aaa"));
Console.WriteLine(checkPalindrome("abc"));
Console.WriteLine(checkPalindrome("madam"));
Console.WriteLine(checkPalindrome("1234"));
}
}
Sample Output:
True False True False
Pictorial Presentation:
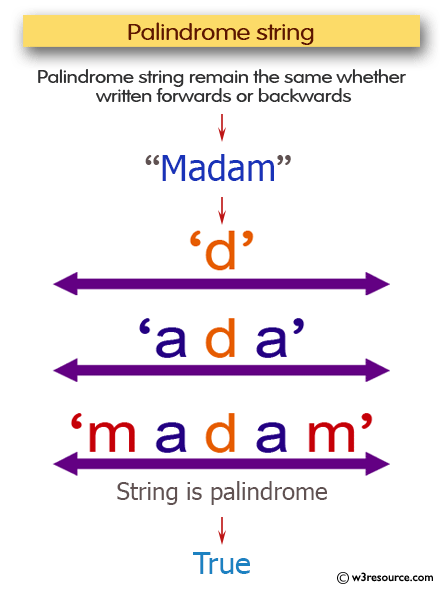
Flowchart:

C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# program to find the pair of adjacent elements that has the largest product of an given array.
Next: Write a C# program to find the pair of adjacent elements that has the highest product of an given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework