C# Sharp Exercises: Find the pair of adjacent elements that has the largest product of an given array which is equal to a given value
C# Sharp Basic: Exercise-55 with Solution
Write a C# program to find the pair of adjacent elements that has the largest product of an given array which is equal to a given value.
Sample Solution:
C# Sharp Code:
using System;
public class Exercise
{
public static int array_adjacent_elements_product(int[] input_array)
{
int array_index = 0;
int product = input_array[array_index] * input_array[array_index + 1];
array_index++;
while (array_index + 1 < input_array.Length)
{
product = ((input_array[array_index] * input_array[array_index + 1]) > product) ?
(input_array[array_index] * input_array[array_index + 1]) :
product;
array_index++;
}
return product;
}
public static void Main()
{
Console.WriteLine(array_adjacent_elements_product(new int[] { 2, 4, 2, 6, 9, 3 }) == 27);
Console.WriteLine(array_adjacent_elements_product(new int[] { 0, -1,-1, -2 }) == 2);
Console.WriteLine(array_adjacent_elements_product(new int[] { 6, 1, 12, 3, 1, 4 }) == 36);
Console.WriteLine(array_adjacent_elements_product(new int[] { 1, 4, 3, 0 }) == 16);
}
}
Sample Output:
False True True False
Flowchart:
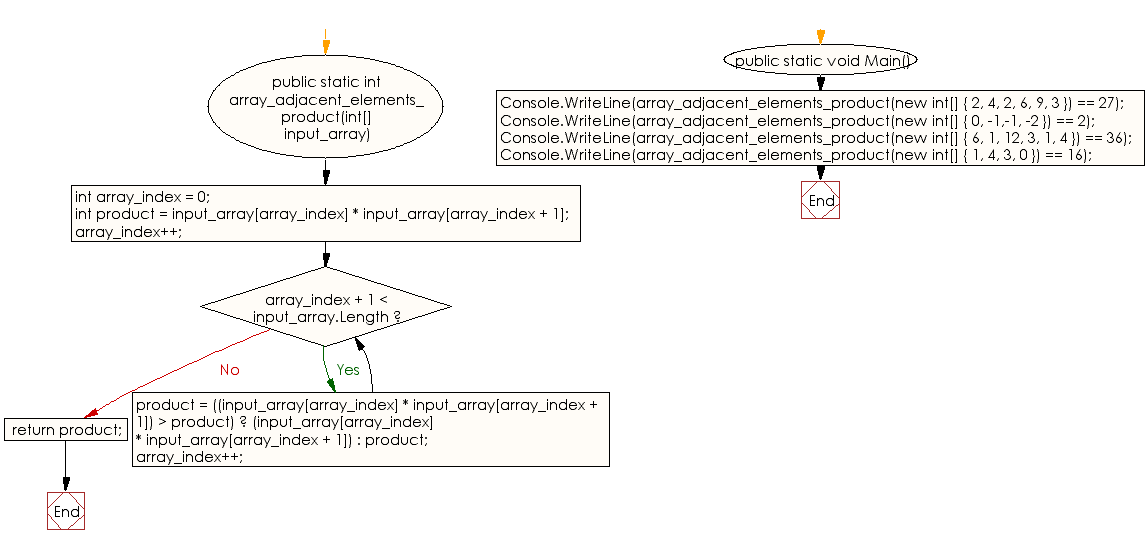
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# program to get the century from a year.
Next: Write a C# program to check if a given string is a palindrome or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework