C Exercises: Check whether a given number is Deficient or not
C Numbers: Exercise-4 with Solution
Write a program in C to check whether a given number is Deficient or not.
Test Data
Input an integer number: 15
Sample Solution:
C Code:
# include <stdio.h>
# include <stdbool.h>
# include <math.h>
int getSum(int n)
{
int sum = 0;
for (int i=1; i<=sqrt(n); i++)
{
if (n%i==0)
{
if (n/i == i)
sum = sum + i;
else
{
sum = sum + i;
sum = sum + (n / i);
}
}
}
sum = sum - n;
return sum;
}
bool checkDeficient(int n)
{
return (getSum(n) < n);
}
int main()
{
int n;
printf("\n\n Check whether a given number is Deficient or not:\n");
printf(" -----------------------------------------------------\n");
printf(" Input an integer number: ");
scanf("%d",&n);
checkDeficient(n)? printf(" The number is Deficient.\n") : printf(" The number is not Deficient.\n");
return 0;
}
Sample Output:
Input an integer number: 15 The number is Deficient.
Pictorial Presentation:
Flowchart:
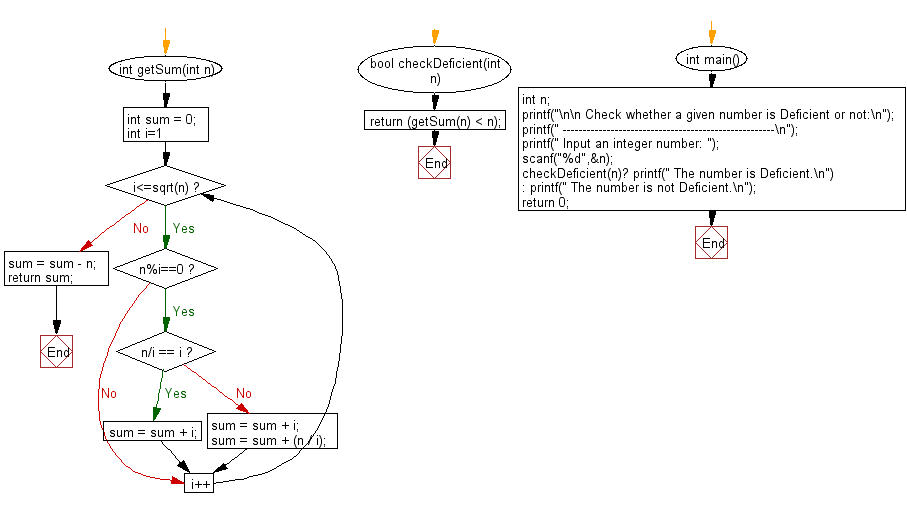
C Programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to find the Abundant numbers (integers) between 1 to 1000.
Next: Write a program in C to find the Deficient numbers (integers) between 1 to 100.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
C Programming: Tips of the Day
Static variable inside of a function in C
The scope of variable is where the variable name can be seen. Here, x is visible only inside function foo().
The lifetime of a variable is the period over which it exists. If x were defined without the keyword static, the lifetime would be from the entry into foo() to the return from foo(); so it would be re-initialized to 5 on every call.
The keyword static acts to extend the lifetime of a variable to the lifetime of the programme; e.g. initialization occurs once and once only and then the variable retains its value - whatever it has come to be - over all future calls to foo().
Ref : https://bit.ly/3fOq7XP
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework