C Exercises: Convert a given amount to possible number of notes and coins
C Basic Declarations and Expressions: Exercise-100 with Solution
Write a C program to convert a currency value (floating point with two decimal places) to possible number of notes and coins.
Possible Notes: 100, 50, 20, 10, 5, 2, 1
Possible Coins: 0.50, 0.25, 0.10, 0.05 and 0.01
Sample Solution:
C Code:
#include <stdio.h>
#include <math.h>
int main() {
double amt;
unsigned int int_amt, frac_amt;
printf("Input the currency value (floating point with two decimal places):\n");
scanf("%lf", &amt);
int_amt = (int) amt;
amt -= int_amt;
frac_amt = round((amt * 100));
printf("\nCurrency Notes:");
printf("\n100 number of Note(s): %d", int_amt / 100);
int_amt -= (int_amt / 100) * 100;
if (int_amt > 50) {
printf("\n50 number of Note(s): 1");
int_amt -= 50;
}
if (int_amt/20 > 0)
printf("\n20 number of Note(s): %d", int_amt / 20);
int_amt -= (int_amt / 20) * 20;
if (int_amt/10 > 0)
printf("\n10 number of Note(s): %d", int_amt / 10);
int_amt -= (int_amt / 10) * 10;
if (int_amt/5 > 0)
printf("\n5 number of Note(s): %d", int_amt / 5);
int_amt -= (int_amt / 5) * 5;
if (int_amt > 0)
printf("\n2 number of Note(s): %d", int_amt / 2);
int_amt -= (int_amt / 2) * 2;
if (int_amt > 0)
printf("\n1 number of Note(s): %d", int_amt);
printf("\n\nCurrency Coins:");
if (frac_amt > 50) {
printf("\n.50 number of Coin(s): 1");
frac_amt -= 50;
}
if (frac_amt/25 > 0)
printf("\n.25 number of Coin(s): %d", frac_amt / 25);
frac_amt -= (frac_amt / 25) * 25;
if (frac_amt/10 > 0)
printf("\n.10 number of Coin(s): %d", frac_amt / 10);
frac_amt -= (frac_amt / 10) * 10;
if (frac_amt/5 > 0)
printf("\n.05 number of Coin(s): %d", frac_amt / 5);
frac_amt -= (frac_amt / 5) * 5;
if (frac_amt > 0)
printf("\n.01 number of Coin(s): %d", frac_amt);
return 0;
}
Sample Output:
Input the currency value (floating point with two decimal places): 10387.75 Currency Notes: 100 number of Note(s): 103 50 number of Note(s): 1 20 number of Note(s): 1 10 number of Note(s): 1 5 number of Note(s): 1 2 number of Note(s): 1 Currency Coins: .50 number of Coin(s): 1 .25 number of Coin(s): 1
Flowchart:
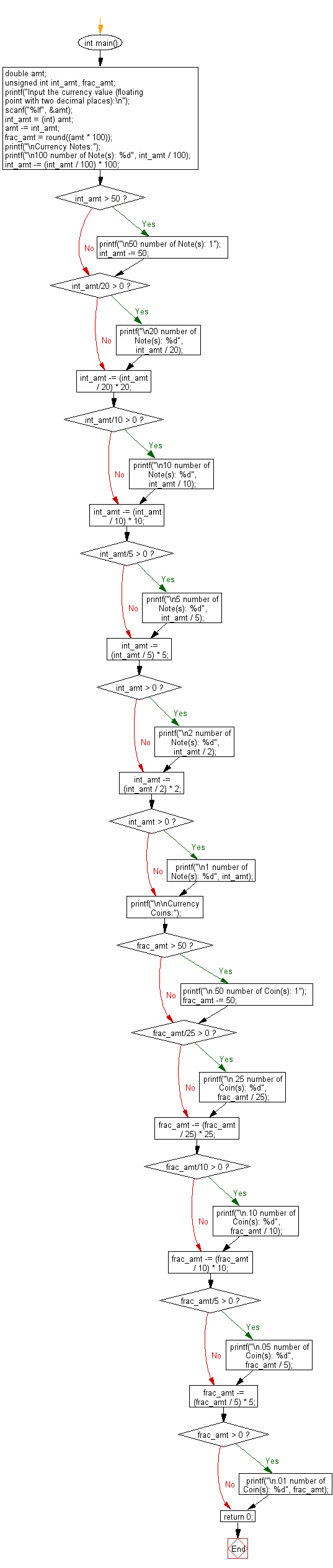
C programming Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C program that takes some integer values from the user and print a histogram.
Next: There are three given ranges, write a C program that reads a floating-point number and find the range where it belongs from four given ranges.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
C Programming: Tips of the Day
Static variable inside of a function in C
The scope of variable is where the variable name can be seen. Here, x is visible only inside function foo().
The lifetime of a variable is the period over which it exists. If x were defined without the keyword static, the lifetime would be from the entry into foo() to the return from foo(); so it would be re-initialized to 5 on every call.
The keyword static acts to extend the lifetime of a variable to the lifetime of the programme; e.g. initialization occurs once and once only and then the variable retains its value - whatever it has come to be - over all future calls to foo().
Ref : https://bit.ly/3fOq7XP
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework