SQLite Exercise: Find the employee id, name along with their manager_id, manager name
Write a query to find the employee id, name (last_name) along with their manager_id, manager name (last_name).
Sample table : employees
SQLite Code:
SELECT e.employee_id 'Emp_Id', e.last_name 'Employee',
m.employee_id 'Mgr_Id', m.last_name 'Manager'
FROM employees e
join employees m
ON (e.manager_id = m.employee_id);
Output:
Emp_Id Employee Mgr_Id Manager ---------- ---------- ---------- ---------- 101 Kochhar 100 King 102 De Haan 100 King 103 Hunold 102 De Haan 104 Ernst 103 Hunold 105 Austin 103 Hunold 106 Pataballa 103 Hunold 107 Lorentz 103 Hunold 108 Greenberg 101 Kochhar 109 Faviet 108 Greenberg 110 Chen 108 Greenberg 111 Sciarra 108 Greenberg 112 Urman 108 Greenberg 113 Popp 108 Greenberg 114 Raphaely 100 King 115 Khoo 114 Raphaely 116 Baida 114 Raphaely 117 Tobias 114 Raphaely 118 Himuro 114 Raphaely 119 Colmenares 114 Raphaely 120 Weiss 100 King 121 Fripp 100 King 122 Kaufling 100 King 123 Vollman 100 King 124 Mourgos 100 King 125 Nayer 120 Weiss 126 Mikkilinen 120 Weiss 127 Landry 120 Weiss 128 Markle 120 Weiss 129 Bissot 121 Fripp 130 Atkinson 121 Fripp 131 Marlow 121 Fripp 132 Olson 121 Fripp 133 Mallin 122 Kaufling 134 Rogers 122 Kaufling 135 Gee 122 Kaufling 136 Philtanker 122 Kaufling 137 Ladwig 123 Vollman 138 Stiles 123 Vollman 139 Seo 123 Vollman 140 Patel 123 Vollman 141 Rajs 124 Mourgos 142 Davies 124 Mourgos 143 Matos 124 Mourgos 144 Vargas 124 Mourgos 145 Russell 100 King 146 Partners 100 King 147 Errazuriz 100 King 148 Cambrault 100 King 149 Zlotkey 100 King 150 Tucker 145 Russell 151 Bernstein 145 Russell 152 Hall 145 Russell 153 Olsen 145 Russell 154 Cambrault 145 Russell 155 Tuvault 145 Russell 156 King 146 Partners 157 Sully 146 Partners 158 McEwen 146 Partners 159 Smith 146 Partners 160 Doran 146 Partners 161 Sewall 146 Partners 162 Vishney 147 Errazuriz 163 Greene 147 Errazuriz 164 Marvins 147 Errazuriz 165 Lee 147 Errazuriz 166 Ande 147 Errazuriz 167 Banda 147 Errazuriz 168 Ozer 148 Cambrault 169 Bloom 148 Cambrault 170 Fox 148 Cambrault 171 Smith 148 Cambrault 172 Bates 148 Cambrault 173 Kumar 148 Cambrault 174 Abel 149 Zlotkey 175 Hutton 149 Zlotkey 176 Taylor 149 Zlotkey 177 Livingston 149 Zlotkey 178 Grant 149 Zlotkey 179 Johnson 149 Zlotkey 180 Taylor 120 Weiss 181 Fleaur 120 Weiss 182 Sullivan 120 Weiss 183 Geoni 120 Weiss 184 Sarchand 121 Fripp 185 Bull 121 Fripp 186 Dellinger 121 Fripp 187 Cabrio 121 Fripp 188 Chung 122 Kaufling 189 Dilly 122 Kaufling 190 Gates 122 Kaufling 191 Perkins 122 Kaufling 192 Bell 123 Vollman 193 Everett 123 Vollman 194 McCain 123 Vollman 195 Jones 123 Vollman 196 Walsh 124 Mourgos 197 Feeney 124 Mourgos 198 OConnell 124 Mourgos 199 Grant 124 Mourgos 200 Whalen 101 Kochhar 201 Hartstein 100 King 202 Fay 201 Hartstein 203 Mavris 101 Kochhar 204 Baer 101 Kochhar 205 Higgins 101 Kochhar 206 Gietz 205 Higgins
Practice SQLite Online
Model Database
Structure of 'hr' database :
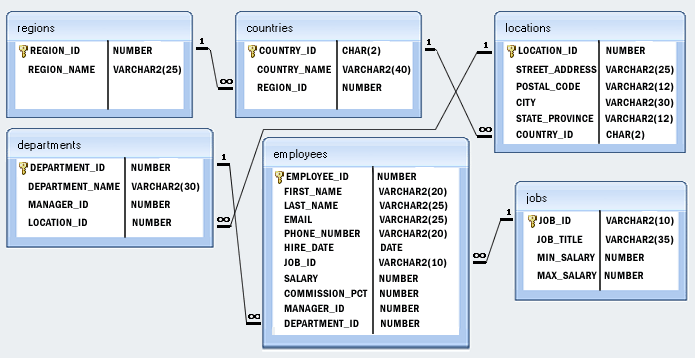
Improve this sample solution and post your code through Disqus.
Previous: Write a query to find the names (first_name, last name), department ID and the name of all the employees.
Next: Write a query to find the names (first_name, last_name) and hire date of the employees who were hired after 'Jones'.
What is the difficulty level of this exercise?
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework