Python: List all files in a directory in Python
Python Basic: Exercise-49 with Solution
Write a Python program to list all files in a directory in Python.
Sample Solution:-
Python Code:
from os import listdir
from os.path import isfile, join
files_list = [f for f in listdir('/home/students') if isfile(join('/home/students', f))]
print(files_list);
Sample Output:
['3ff44d80-2423-11e7-807b-bd9de91b1602.py', 'f5272c90-2423-11e7-807b-bd9de91b1602.py', 'test.txt', 'f34e26d0-2 423-11e7-807b-bd9de91b1602.py', 'f6a62b70-2423-11e7-807b-bd9de91b1602.py', '.mysql_history', '.bash_logout', ' 037db660-240b-11e7-807b-bd9de91b1602.py', '3d299190-241f-11e7-807b-bd9de91b1602.py', '8e7e22f0-240f-11e7-807b- bd9de91b1602.py', '.bash_history', '276b1dd0-2404-11e7-807b-bd9de91b1602.py', '7e2f05d0-241a-11e7-807b-bd9de91 b1602.py', '6956ba40-2429-11e7-807b-bd9de91b1602.py', '.profile', 'abc.py', 'f69a4490-2423-11e7-807b-bd9de91b1 602.py', '.viminfo', 'mynewtest.txt', 'myfile.txt', 'logging_example.out', '.web-term.json', 'd2c942f0-2423-11 e7-807b-bd9de91b1602.py', 'ca7c2750-242a-11e7-807b-bd9de91b1602.py', 'abc.txt', 'exercise.cs', '.bashrc', 'Exa mple.cs', '2f5aa190-2428-11e7-807b-bd9de91b1602.py', '2d5d6540-2418-11e7-807b-bd9de91b1602.py', '5bfc84e0-240d -11e7-807b-bd9de91b1602.py', '940ca5b0-2406-11e7-807b-bd9de91b1602.py', 'myfig.png', 'file.out', 'a7af6660-241 6-11e7-807b-bd9de91b1602.py', '4a690da0-2428-11e7-807b-bd9de91b1602.py', 'line.gif', 'mmm.txt\n', 'temp.txt', 'fb6e28e0-2425-11e7-807b-bd9de91b1602.py', 'f0896180-2423-11e7-807b-bd9de91b1602.py', 'dddd.txt\n', '700da350- 2413-11e7-807b-bd9de91b1602.py', 'sss.dat\n', '05f2fa20-2421-11e7-807b-bd9de91b1602.py', 'result.txt', '68c38d e0-241c-11e7-807b-bd9de91b1602.py', 'output.jpg', 'c930d080-2407-11e7-807b-bd9de91b1602.py', '2e8ace70-2428-11 e7-807b-bd9de91b1602.py', '26492-1274250701.png', 'mytest.txt', '07fd1540-2411-11e7-807b-bd9de91b1602.py']
Flowchart:
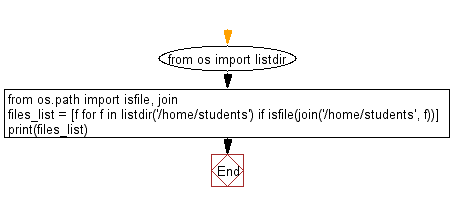
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to parse a string to Float or Integer.
Next: Write a Python program to print without newline or space?
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Python: Tips of the Day
Find current directory and file's directory:
To get the full path to the directory a Python file is contained in, write this in that file:
import os dir_path = os.path.dirname(os.path.realpath(__file__))
(Note that the incantation above won't work if you've already used os.chdir() to change your current working directory, since the value of the __file__ constant is relative to the current working directory and is not changed by an os.chdir() call.)
To get the current working directory use
import os cwd = os.getcwd()
Documentation references for the modules, constants and functions used above:
- The os and os.path modules.
- The __file__ constant
- os.path.realpath(path) (returns "the canonical path of the specified filename, eliminating any symbolic links encountered in the path")
- os.path.dirname(path) (returns "the directory name of pathname path")
- os.getcwd() (returns "a string representing the current working directory")
- os.chdir(path) ("change the current working directory to path")
Ref: https://bit.ly/3fy0R6m
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework