Python Bisect: Find three integers which gives the sum of zero in a given array of integers using Binary Search
Python Bisect: Exercise-7 with Solution
Write a Python program to find three integers which gives the sum of zero in a given array of integers using Binary Search (bisect).
Sample Solution:
Python Code:
from bisect import bisect, bisect_left
from collections import Counter
class Solution:
def three_Sum(self, nums):
"""
:type nums: List[int]
:rtype: List[List[int]]
"""
triplets = []
if len(nums) < 3:
return triplets
num_freq = Counter(nums)
nums = sorted(num_freq) # Sorted unique numbers
max_num = nums[-1]
for i, v in enumerate(nums):
if num_freq[v] >= 2:
complement = -2 * v
if complement in num_freq:
if complement != v or num_freq[v] >= 3:
triplets.append([v] * 2 + [complement])
# When all 3 numbers are different.
if v < 0: # Only when v is the smallest
two_sum = -v
# Lower/upper bound of the smaller of remainingtwo.
lb = bisect_left(nums, two_sum - max_num, i + 1)
ub = bisect(nums, two_sum // 2, lb)
for u in nums[lb : ub]:
complement = two_sum - u
if complement in num_freq and u != complement:
triplets.append([v, u, complement])
return triplets
nums = [-20, 0, 20, 40, -20, -40, 80]
s = Solution()
result = s.three_Sum(nums)
print(result)
nums = [1, 2, 3, 4, 5, -6]
result = s.three_Sum(nums)
print(result)
Sample Output:
[[-40, 0, 40], [-20, -20, 40], [-20, 0, 20]] [[-6, 1, 5], [-6, 2, 4]]
Flowchart:
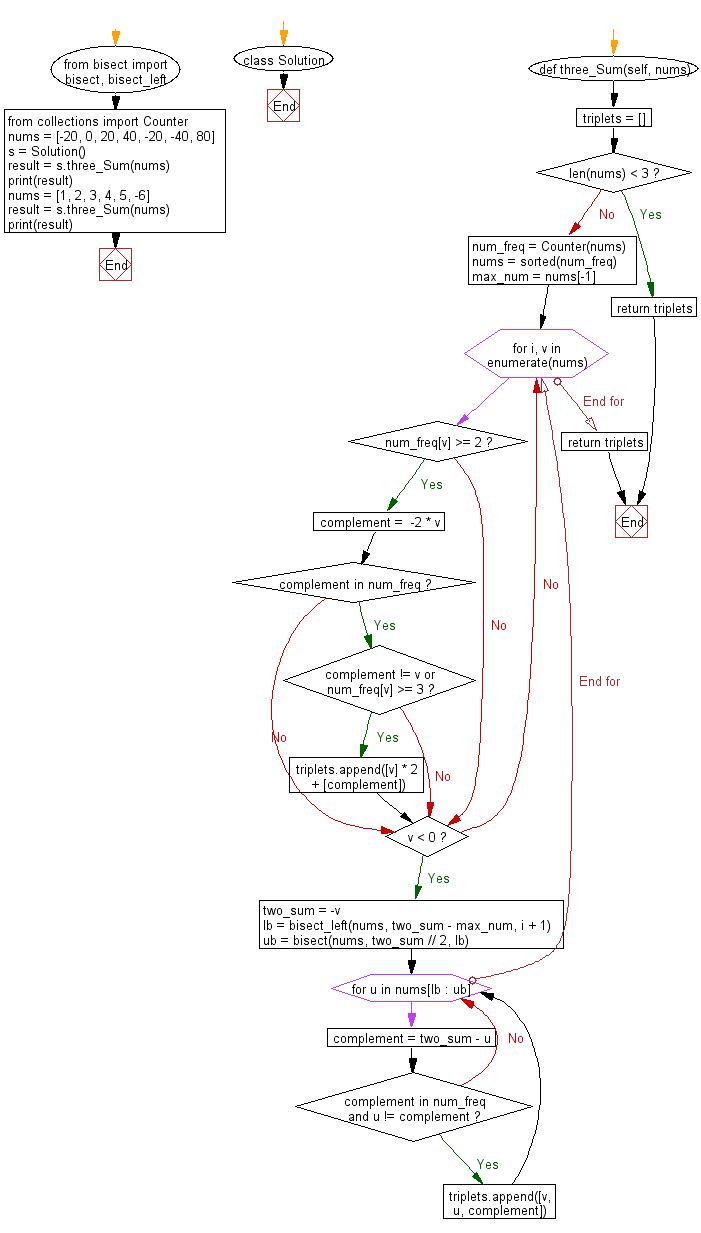
Visualize Python code execution:
The following tool visualize what the computer is doing step-by-step as it executes the said program:
Python Code Editor:
Contribute your code and comments through Disqus.
Next: Write a Python program to find a triplet in an array such that the sum is closest to a given number. Return the sum of the three integers.What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Python: Tips of the Day
Find current directory and file's directory:
To get the full path to the directory a Python file is contained in, write this in that file:
import os dir_path = os.path.dirname(os.path.realpath(__file__))
(Note that the incantation above won't work if you've already used os.chdir() to change your current working directory, since the value of the __file__ constant is relative to the current working directory and is not changed by an os.chdir() call.)
To get the current working directory use
import os cwd = os.getcwd()
Documentation references for the modules, constants and functions used above:
- The os and os.path modules.
- The __file__ constant
- os.path.realpath(path) (returns "the canonical path of the specified filename, eliminating any symbolic links encountered in the path")
- os.path.dirname(path) (returns "the directory name of pathname path")
- os.getcwd() (returns "a string representing the current working directory")
- os.chdir(path) ("change the current working directory to path")
Ref: https://bit.ly/3fy0R6m
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework