Python Projects: Python project to get CO2 emission data from the UK Carbon Intensity API
Python Web Project-1 with Solution
National Grid ESO, in partnership with Environmental Defense Fund Europe, University of Oxford Department of Computer Science and WWF, have developed the world's first Carbon Intensity forecast with a regional breakdown.
The Carbon Intensity API uses state-of-the-art Machine Learning and sophisticated power system modeling to forecast the carbon intensity and generation mix 96+ hours ahead for each region in Great Britain.
This OpenAPI allows consumers and smart devices to schedule and minimize CO2 emissions at a local level.
Source: https://carbonintensity.org.uk/
Create a Python project to get CO2 emission data from the UK Carbon Intensity API.
Sample Json data:
{ "data":[{ "from": "2021-01-24T08:00Z", "to": "2021-01-24T08:30Z", "intensity": { "forecast": 296, "actual": null, "index": "high" } }] }
Sample Solution:
Python Code:
#shorturl.at/hjpwJ
from datetime import date
import requests
BASE_URL = "https://api.carbonintensity.org.uk/intensity"
# Emission in the last half hour
def fetch_last_half_hour() -> str:
last_half_hour = requests.get(BASE_URL).json()["data"][0]
return last_half_hour["intensity"]["actual"]
# Emissions in a specific date range
def fetch_from_to(start, end) -> list:
return requests.get(f"{BASE_URL}/{start}/{end}").json()["data"]
if __name__ == "__main__":
for entry in fetch_from_to(start=date(2020, 10, 1), end=date(2020, 10, 3)):
print("From {from} to {to}: {intensity[actual]}".format(**entry))
Sample Output:
From 2020-09-30T23:30Z to 2020-10-01T00:00Z: 160 From 2020-10-01T00:00Z to 2020-10-01T00:30Z: 164 From 2020-10-01T00:30Z to 2020-10-01T01:00Z: 163 From 2020-10-01T01:00Z to 2020-10-01T01:30Z: 165 From 2020-10-01T01:30Z to 2020-10-01T02:00Z: 167 From 2020-10-01T02:00Z to 2020-10-01T02:30Z: 169 From 2020-10-01T02:30Z to 2020-10-01T03:00Z: 166 From 2020-10-01T03:00Z to 2020-10-01T03:30Z: 174 From 2020-10-01T03:30Z to 2020-10-01T04:00Z: 188 From 2020-10-01T04:00Z to 2020-10-01T04:30Z: 217 From 2020-10-01T04:30Z to 2020-10-01T05:00Z: 239 From 2020-10-01T05:00Z to 2020-10-01T05:30Z: 262 From 2020-10-01T05:30Z to 2020-10-01T06:00Z: 275 From 2020-10-01T06:00Z to 2020-10-01T06:30Z: 289 From 2020-10-01T06:30Z to 2020-10-01T07:00Z: 284 From 2020-10-01T07:00Z to 2020-10-01T07:30Z: 276 From 2020-10-01T07:30Z to 2020-10-01T08:00Z: 272 From 2020-10-01T08:00Z to 2020-10-01T08:30Z: 267 From 2020-10-01T08:30Z to 2020-10-01T09:00Z: 266 From 2020-10-01T09:00Z to 2020-10-01T09:30Z: 260 From 2020-10-01T09:30Z to 2020-10-01T10:00Z: 254 From 2020-10-01T10:00Z to 2020-10-01T10:30Z: 250 From 2020-10-01T10:30Z to 2020-10-01T11:00Z: 247 From 2020-10-01T11:00Z to 2020-10-01T11:30Z: 242 From 2020-10-01T11:30Z to 2020-10-01T12:00Z: 242 From 2020-10-01T12:00Z to 2020-10-01T12:30Z: 239 From 2020-10-01T12:30Z to 2020-10-01T13:00Z: 236 From 2020-10-01T13:00Z to 2020-10-01T13:30Z: 236 From 2020-10-01T13:30Z to 2020-10-01T14:00Z: 250 From 2020-10-01T14:00Z to 2020-10-01T14:30Z: 268 From 2020-10-01T14:30Z to 2020-10-01T15:00Z: 277 From 2020-10-01T15:00Z to 2020-10-01T15:30Z: 291 From 2020-10-01T15:30Z to 2020-10-01T16:00Z: 302 From 2020-10-01T16:00Z to 2020-10-01T16:30Z: 306 From 2020-10-01T16:30Z to 2020-10-01T17:00Z: 305 From 2020-10-01T17:00Z to 2020-10-01T17:30Z: 307 From 2020-10-01T17:30Z to 2020-10-01T18:00Z: 308 From 2020-10-01T18:00Z to 2020-10-01T18:30Z: 308 From 2020-10-01T18:30Z to 2020-10-01T19:00Z: 303 From 2020-10-01T19:00Z to 2020-10-01T19:30Z: 294 From 2020-10-01T19:30Z to 2020-10-01T20:00Z: 283 From 2020-10-01T20:00Z to 2020-10-01T20:30Z: 267 From 2020-10-01T20:30Z to 2020-10-01T21:00Z: 262 From 2020-10-01T21:00Z to 2020-10-01T21:30Z: 229 From 2020-10-01T21:30Z to 2020-10-01T22:00Z: 209 From 2020-10-01T22:00Z to 2020-10-01T22:30Z: 199 From 2020-10-01T22:30Z to 2020-10-01T23:00Z: 189 From 2020-10-01T23:00Z to 2020-10-01T23:30Z: 175 From 2020-10-01T23:30Z to 2020-10-02T00:00Z: 163 From 2020-10-02T00:00Z to 2020-10-02T00:30Z: 160 From 2020-10-02T00:30Z to 2020-10-02T01:00Z: 160 From 2020-10-02T01:00Z to 2020-10-02T01:30Z: 150 From 2020-10-02T01:30Z to 2020-10-02T02:00Z: 147 From 2020-10-02T02:00Z to 2020-10-02T02:30Z: 141 From 2020-10-02T02:30Z to 2020-10-02T03:00Z: 154 From 2020-10-02T03:00Z to 2020-10-02T03:30Z: 147 From 2020-10-02T03:30Z to 2020-10-02T04:00Z: 154 From 2020-10-02T04:00Z to 2020-10-02T04:30Z: 160 From 2020-10-02T04:30Z to 2020-10-02T05:00Z: 166 From 2020-10-02T05:00Z to 2020-10-02T05:30Z: 182 From 2020-10-02T05:30Z to 2020-10-02T06:00Z: 191 From 2020-10-02T06:00Z to 2020-10-02T06:30Z: 193 From 2020-10-02T06:30Z to 2020-10-02T07:00Z: 186 From 2020-10-02T07:00Z to 2020-10-02T07:30Z: 180 From 2020-10-02T07:30Z to 2020-10-02T08:00Z: 178 From 2020-10-02T08:00Z to 2020-10-02T08:30Z: 181 From 2020-10-02T08:30Z to 2020-10-02T09:00Z: 178 From 2020-10-02T09:00Z to 2020-10-02T09:30Z: 177 From 2020-10-02T09:30Z to 2020-10-02T10:00Z: 176 From 2020-10-02T10:00Z to 2020-10-02T10:30Z: 166 From 2020-10-02T10:30Z to 2020-10-02T11:00Z: 169 From 2020-10-02T11:00Z to 2020-10-02T11:30Z: 171 From 2020-10-02T11:30Z to 2020-10-02T12:00Z: 172 From 2020-10-02T12:00Z to 2020-10-02T12:30Z: 170 From 2020-10-02T12:30Z to 2020-10-02T13:00Z: 171 From 2020-10-02T13:00Z to 2020-10-02T13:30Z: 170 From 2020-10-02T13:30Z to 2020-10-02T14:00Z: 162 From 2020-10-02T14:00Z to 2020-10-02T14:30Z: 165 From 2020-10-02T14:30Z to 2020-10-02T15:00Z: 168 From 2020-10-02T15:00Z to 2020-10-02T15:30Z: 176 From 2020-10-02T15:30Z to 2020-10-02T16:00Z: 181 From 2020-10-02T16:00Z to 2020-10-02T16:30Z: 183 From 2020-10-02T16:30Z to 2020-10-02T17:00Z: 177 From 2020-10-02T17:00Z to 2020-10-02T17:30Z: 176 From 2020-10-02T17:30Z to 2020-10-02T18:00Z: 175 From 2020-10-02T18:00Z to 2020-10-02T18:30Z: 172 From 2020-10-02T18:30Z to 2020-10-02T19:00Z: 171 From 2020-10-02T19:00Z to 2020-10-02T19:30Z: 162 From 2020-10-02T19:30Z to 2020-10-02T20:00Z: 152 From 2020-10-02T20:00Z to 2020-10-02T20:30Z: 146 From 2020-10-02T20:30Z to 2020-10-02T21:00Z: 162 From 2020-10-02T21:00Z to 2020-10-02T21:30Z: 135 From 2020-10-02T21:30Z to 2020-10-02T22:00Z: 124 From 2020-10-02T22:00Z to 2020-10-02T22:30Z: 110 From 2020-10-02T22:30Z to 2020-10-02T23:00Z: 104 From 2020-10-02T23:00Z to 2020-10-02T23:30Z: 97 From 2020-10-02T23:30Z to 2020-10-03T00:00Z: 102
Flowchart:
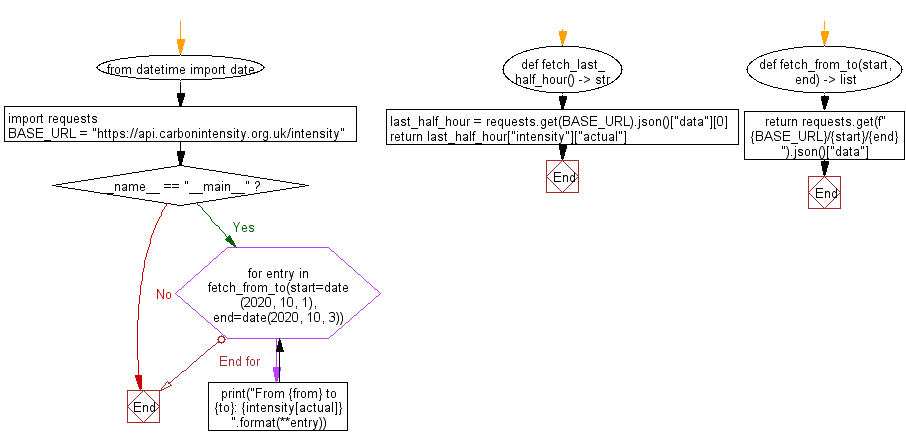
Improve this sample solutions and post your code through Disqus
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework