PHP Searching and Sorting Algorithm: Gnome sort
PHP Searching and Sorting Algorithm: Exercise-9 with Solution
Write a PHP program to sort a list of elements using Gnome sort.
Gnome sort is a sorting algorithm originally proposed by Dr. Hamid Sarbazi-Azad (Professor of Computer Engineering at Sharif University of Technology) in 2000 and called "stupid sort" (not to be confused with bogosort), and then later on described by Dick Grune and named "gnome sort".
The algorithm always finds the first place where two adjacent elements are in the wrong order and swaps them. It takes advantage of the fact that performing a swap can introduce a new out-of-order adjacent pair only next to the two swapped elements.
A visualization of the gnome sort :
Sample Solution :
PHP Code :
<?php
function gnome_Sort($my_array){
$i = 1;
$j = 2;
while($i < count($my_array)){
if ($my_array[$i-1] <= $my_array[$i]){
$i = $j;
$j++;
}else{
list($my_array[$i],$my_array[$i-1]) = array($my_array[$i-1],$my_array[$i]);
$i--;
if($i == 0){
$i = $j;
$j++;
}
}
}
return $my_array;
}
$test_array = array(3, 0, 2, 5, -1, 4, 1);
echo "Original Array :\n";
echo implode(', ',$test_array );
echo "\nSorted Array :\n";
echo implode(', ',gnome_Sort($test_array)). PHP_EOL;
?>
Sample Output:
3, 0, 2, 5, -1, 4, 1 Sorted Array : -1, 0, 1, 2, 3, 4, 5
Flowchart :
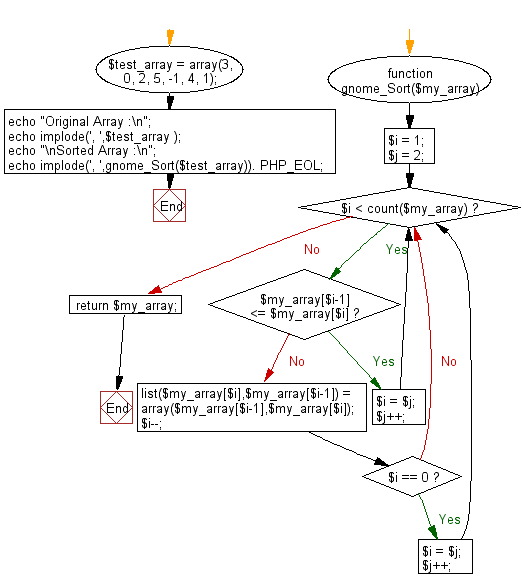
PHP Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a PHP program to sort a list of elements using Comb sort.
Next: Write a PHP program to sort a list of elements using Bucket sort.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
PHP: Tips of the Day
How to Sort Multi-dimensional Array by Value?
Try a usort, If you are still on PHP 5.2 or earlier, you'll have to define a sorting function first:
Example:
function sortByOrder($a, $b) { return $a['order'] - $b['order']; } usort($myArray, 'sortByOrder');
Starting in PHP 5.3, you can use an anonymous function:
usort($myArray, function($a, $b) { return $a['order'] - $b['order']; });
And finally with PHP 7 you can use the spaceship operator:
usort($myArray, function($a, $b) { return $a['order'] <=> $b['order']; });
To extend this to multi-dimensional sorting, reference the second/third sorting elements if the first is zero - best explained below. You can also use this for sorting on sub-elements.
usort($myArray, function($a, $b) { $retval = $a['order'] <=> $b['order']; if ($retval == 0) { $retval = $a['suborder'] <=> $b['suborder']; if ($retval == 0) { $retval = $a['details']['subsuborder'] <=> $b['details']['subsuborder']; } } return $retval; });
If you need to retain key associations, use uasort() - see comparison of array sorting functions in the manual
Ref : https://bit.ly/3i77vCC
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework