PHP Exercises: Find out the maximum element between the first or last element in a given array of integers, replace all elements with maximum element
PHP Basic Algorithm: Exercise-92 with Solution
Write a PHP program to find out the maximum element between the first or last element in a given array of integers ( length 4), replace all elements with maximum element.
Sample Solution:
PHP Code :
<?php
function test($nums)
{
$max = max($nums);
return [$max, $max, $max, $max];
}
$a = [10, 20, -30, -40];
echo "Original array: " . implode(',', $a) . "\n";
$result = test($a);
echo "New array with maximum values: " . implode(',', $result);
Sample Output:
Original array: 10,20,-30,-40 New array with maximum values: 20,20,20,20
Flowchart:
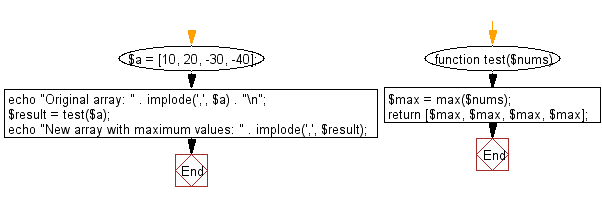
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to reverse a given array of integers and length 5.
Next: Write a PHP program to create a new array containing the middle elements from the two given arrays of integers, each length 5.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
PHP: Tips of the Day
How to Sort Multi-dimensional Array by Value?
Try a usort, If you are still on PHP 5.2 or earlier, you'll have to define a sorting function first:
Example:
function sortByOrder($a, $b) { return $a['order'] - $b['order']; } usort($myArray, 'sortByOrder');
Starting in PHP 5.3, you can use an anonymous function:
usort($myArray, function($a, $b) { return $a['order'] - $b['order']; });
And finally with PHP 7 you can use the spaceship operator:
usort($myArray, function($a, $b) { return $a['order'] <=> $b['order']; });
To extend this to multi-dimensional sorting, reference the second/third sorting elements if the first is zero - best explained below. You can also use this for sorting on sub-elements.
usort($myArray, function($a, $b) { $retval = $a['order'] <=> $b['order']; if ($retval == 0) { $retval = $a['suborder'] <=> $b['suborder']; if ($retval == 0) { $retval = $a['details']['subsuborder'] <=> $b['details']['subsuborder']; } } return $retval; });
If you need to retain key associations, use uasort() - see comparison of array sorting functions in the manual
Ref : https://bit.ly/3i77vCC
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework