PHP Exercises: Create a new string of length 2 starting at the given index of a given string
PHP Basic Algorithm: Exercise-73 with Solution
Write a PHP program to create a new string of length 2 starting at the given index of a given string.
Sample Solution:
PHP Code :
<?php
function test($s1, $index)
{
return $index + 2 <= strlen($s1) ? substr($s1, $index, 2) : substr($s1, 0, 2);
}
echo test("Hello", 1)."\n";
echo test("Python", 2)."\n";
echo test("on", 1)."\n";
Sample Output:
el th on
Flowchart:
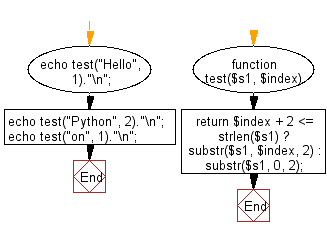
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a PHP program to create a new string using the first and last n characters from a given string of length at least n.
Next: Write a PHP program to create a new string taking 3 characters from the middle of a given string at least 3.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
PHP: Tips of the Day
How to Sort Multi-dimensional Array by Value?
Try a usort, If you are still on PHP 5.2 or earlier, you'll have to define a sorting function first:
Example:
function sortByOrder($a, $b) { return $a['order'] - $b['order']; } usort($myArray, 'sortByOrder');
Starting in PHP 5.3, you can use an anonymous function:
usort($myArray, function($a, $b) { return $a['order'] - $b['order']; });
And finally with PHP 7 you can use the spaceship operator:
usort($myArray, function($a, $b) { return $a['order'] <=> $b['order']; });
To extend this to multi-dimensional sorting, reference the second/third sorting elements if the first is zero - best explained below. You can also use this for sorting on sub-elements.
usort($myArray, function($a, $b) { $retval = $a['order'] <=> $b['order']; if ($retval == 0) { $retval = $a['suborder'] <=> $b['suborder']; if ($retval == 0) { $retval = $a['details']['subsuborder'] <=> $b['details']['subsuborder']; } } return $retval; });
If you need to retain key associations, use uasort() - see comparison of array sorting functions in the manual
Ref : https://bit.ly/3i77vCC
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework