JavaScript: The longest palindrome in a specified string
JavaScript Function: Exercise-27 with Solution
Write a JavaScript function that returns the longest palindrome in a given string.
Note: According to Wikipedia "In computer science, the longest palindromic substring or longest symmetric factor problem is
the problem of finding a maximum-length contiguous substring of a given string that is also a palindrome.
For example, the longest palindromic substring of "bananas" is "anana".
The longest palindromic substring is not guaranteed to be unique; for example,
in the string "abracadabra", there is no palindromic substring with length greater than three,
but there are two palindromic substrings with length three, namely, "aca" and "ada".
In some applications it may be necessary to return all maximal
palindromic substrings (that is, all substrings that are themselves palindromes and cannot
be extended to larger palindromic substrings) rather than returning only one substring or
returning the maximum length of a palindromic substring.
Pictorial Presentation:
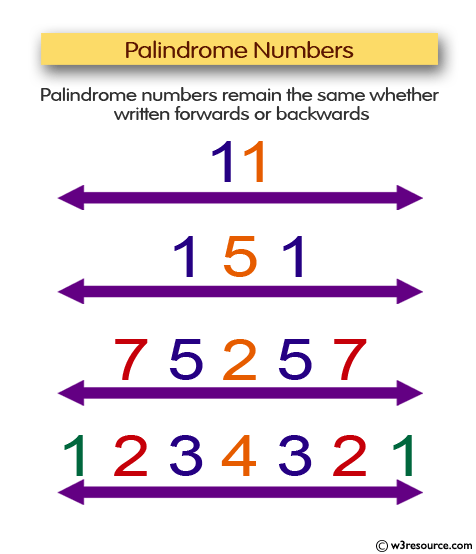
Sample Solution: -
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Write a JavaScript function that returns the longest palindrome in a given string</title>
</head>
<body>
</body>
</html>
JavaScript Code:
function is_Palindrome(str1) {
var rev = str1.split("").reverse().join("");
return str1 == rev;
}
function longest_palindrome(str1){
var max_length = 0,
maxp = '';
for(var i=0; i < str1.length; i++)
{
var subs = str1.substr(i, str1.length);
for(var j=subs.length; j>=0; j--)
{
var sub_subs_str = subs.substr(0, j);
if (sub_subs_str.length <= 1)
continue;
if (is_Palindrome(sub_subs_str))
{
if (sub_subs_str.length > max_length)
{
max_length = sub_subs_str.length;
maxp = sub_subs_str;
}
}
}
}
return maxp;
}
console.log(longest_palindrome("abracadabra"));
console.log(longest_palindrome("HYTBCABADEFGHABCDEDCBAGHTFYW12345678987654321ZWETYGDE"));
Output:
aca 12345678987654321
Flowchart:

Live Demo:
See the Pen JavaScript - The longest palindrome in a specified string-function-ex- 27 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript function to find longest substring in a given a string without repeating characters.
Next: Write a JavaScript program to pass a 'JavaScript function' as parameter.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
JavaScript: Tips of the Day
How to insert an item into an array at a specific index (JavaScript)?
What you want is the splice function on the native array object.
arr.splice(index, 0, item); will insert item into arr at the specified index (deleting 0 items first, that is, it's just an insert). In this example we will create an array and add an element to it into index 2:
var arr = []; arr[0] = "Jani"; arr[1] = "Hege"; arr[2] = "Stale"; arr[3] = "Kai Jim"; arr[4] = "Borge"; console.log(arr.join()); arr.splice(2, 0, "Lene"); console.log(arr.join());
Ref: https://bit.ly/2BXbp04
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework