JavaScript: Highlight the bold words of a paragraph, on mouse over a certain link
JavaScript DOM: Exercise-12 with Solution
Write a JavaScript program to highlight the bold words of the following paragraph, on mouse over a certain link.
Sample link and text:
[On mouse over here bold words of the following paragraph will be highlighted]Sample Solution: -
HTML Code:
<!doctype html>
<html>
<head>
<meta charset="UTF-8">
<title>Get And Style All Tags</title>
</head>
<body>
<p>[<a href="#" onMouseOver="highlight()" onMouseOut="return_normal()">On mouse over here bold words of the following paragraph will be highlighted</a>]</p>
<p><strong>We</strong> have just started <strong>this</strong> section for the users (<strong>beginner</strong> to intermediate) who <strong>want</strong> to work with <strong>various</strong> JavaScript <strong>problems</strong> and write scripts online to <strong>test</strong> their JavaScript <strong>skill</strong>.</p>
</body>
</html>
JavaScript Code:
//First create a list of all bold items
var bold_Items;
window.onload = getBold_items();
// Collect all <strong> tags
function getBold_items()
{
bold_Items = document.getElementsByTagName('strong');
}
// iterate all bold tags and change color
function highlight()
{
for (var i=0; i<bold_Items.length; i++)
{
bold_Items[i].style.color = "green";
}
}
// On mouse out highlighted words become black
function return_normal()
{
for (var i=0; i<bold_Items.length; i++)
{
bold_Items[i].style.color = "black";
}
}
Sample Output:

Flowchart:
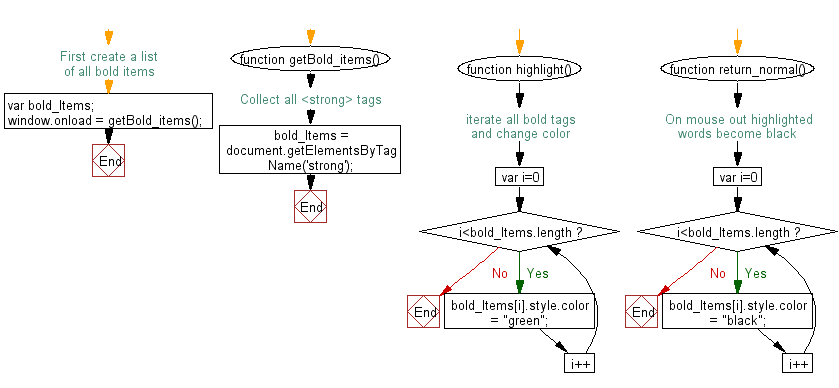
Live Demo:
See the Pen javascript-dom-exercise-12 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to display a random image (clicking on a button) from the following list.
Next: Write a JavaScript program to get the width and height of the window (any time the window is resized).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
JavaScript: Tips of the Day
How to insert an item into an array at a specific index (JavaScript)?
What you want is the splice function on the native array object.
arr.splice(index, 0, item); will insert item into arr at the specified index (deleting 0 items first, that is, it's just an insert). In this example we will create an array and add an element to it into index 2:
var arr = []; arr[0] = "Jani"; arr[1] = "Hege"; arr[2] = "Stale"; arr[3] = "Kai Jim"; arr[4] = "Borge"; console.log(arr.join()); arr.splice(2, 0, "Lene"); console.log(arr.join());
Ref: https://bit.ly/2BXbp04
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework