JavaScript: Compute the sum of two parts and store into an array of size two
JavaScript Basic: Exercise-85 with Solution
Write a JavaScript code to divide a given array of positive integers into two parts. First element goes to first part, second element goes to second part, and third element goes to first part and so on. Now compute the sum of two parts and store into an array of size two.
Pictorial Presentation:
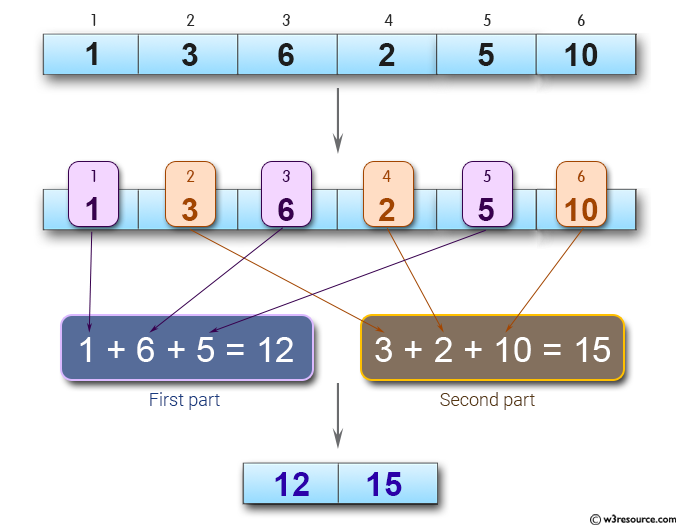
Sample Solution:
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Compute the sum of two parts and store into an array of size two</title>
</head>
<body>
</body>
</html>
JavaScript Code:
function alternate_Sums(arr) {
var result = [0, 0];
for(var i = 0; i < arr.length; i++)
{
if(i % 2) result[1] += arr[i];
else
result[0] += arr[i];
}
return result
}
console.log(alternate_Sums([1, 3, 6, 2, 5, 10]))
Sample Output:
[12,15]
Flowchart:

ES6 Version:
function alternate_Sums(arr) {
const result = [0, 0];
for(let i = 0; i < arr.length; i++)
{
if(i % 2) result[1] += arr[i];
else
result[0] += arr[i];
}
return result
}
console.log(alternate_Sums([1, 3, 6, 2, 5, 10]))
Live Demo:
See the Pen javascript-basic-exercise-85 by w3resource (@w3resource) on CodePen.
Contribute your code and comments through Disqus.
Previous: Write a JavaScript to replace each character of a given string by the next one in the English alphabet.
Next: Write a JavaScript program to find the types of a given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
JavaScript: Tips of the Day
How to insert an item into an array at a specific index (JavaScript)?
What you want is the splice function on the native array object.
arr.splice(index, 0, item); will insert item into arr at the specified index (deleting 0 items first, that is, it's just an insert). In this example we will create an array and add an element to it into index 2:
var arr = []; arr[0] = "Jani"; arr[1] = "Hege"; arr[2] = "Stale"; arr[3] = "Kai Jim"; arr[4] = "Borge"; console.log(arr.join()); arr.splice(2, 0, "Lene"); console.log(arr.join());
Ref: https://bit.ly/2BXbp04
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework