JavaScript: Check from three given numbers that two or all of them have the same rightmost digit
JavaScript Basic: Exercise-43 with Solution
Write a JavaScript program to check from three given numbers (non negative integers) that two or all of them have the same rightmost digit.
Pictorial Presentation:
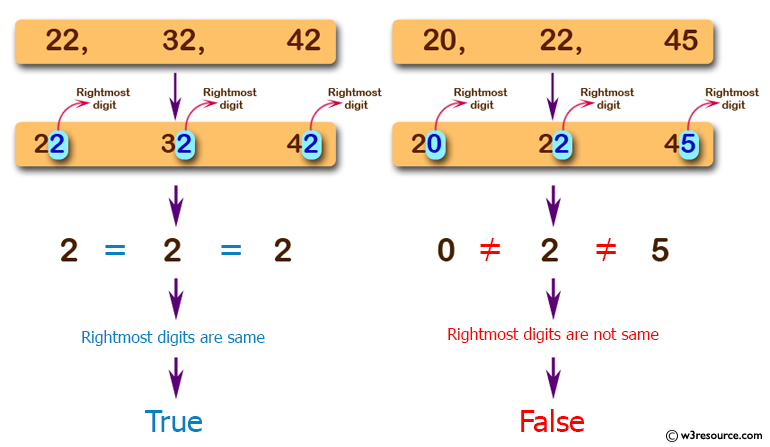
Sample Solution:
HTML Code:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>JavaScript program to check from three given numbers (non negative integers) that two or all of them have the same rightmost digit.</title>
</head>
<body>
</body>
</html>
JavaScript Code:
function same_last_digit(p, q, r) {
return (p % 10 === q % 10) ||
(p % 10 === r % 10) ||
(q % 10 === r % 10);
}
console.log(same_last_digit(22,32,42));
console.log(same_last_digit(102,302,2));
console.log(same_last_digit(20,22,45));
Sample Output:
true true false
Flowchart:
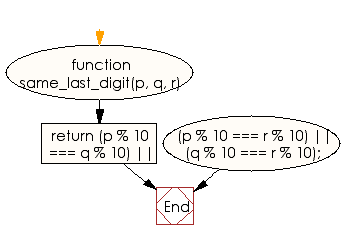
ES6 Version:
function same_last_digit(p, q, r) {
return (p % 10 === q % 10) ||
(p % 10 === r % 10) ||
(q % 10 === r % 10);
}
console.log(same_last_digit(22,32,42));
console.log(same_last_digit(102,302,2));
console.log(same_last_digit(20,22,45));
Live Demo:
See the Pen JavaScript: Check from three given numbers - basic-ex-43 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript program to check if three given numbers are increasing in strict mode or in soft mode.
Next: Write a JavaScript program to check from three given integers that if a number is greater than or equal to 20 and less than one of the others.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
JavaScript: Tips of the Day
How to insert an item into an array at a specific index (JavaScript)?
What you want is the splice function on the native array object.
arr.splice(index, 0, item); will insert item into arr at the specified index (deleting 0 items first, that is, it's just an insert). In this example we will create an array and add an element to it into index 2:
var arr = []; arr[0] = "Jani"; arr[1] = "Hege"; arr[2] = "Stale"; arr[3] = "Kai Jim"; arr[4] = "Borge"; console.log(arr.join()); arr.splice(2, 0, "Lene"); console.log(arr.join());
Ref: https://bit.ly/2BXbp04
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework