JavaScript: Get nth largest element from an unsorted array
JavaScript Array: Exercise-34 with Solution
Write a JavaScript function to get nth largest element from an unsorted array.
Test Data:
console.log(nthlargest([ 43, 56, 23, 89, 88, 90, 99, 652], 4));
89
Pictorial Presentation:
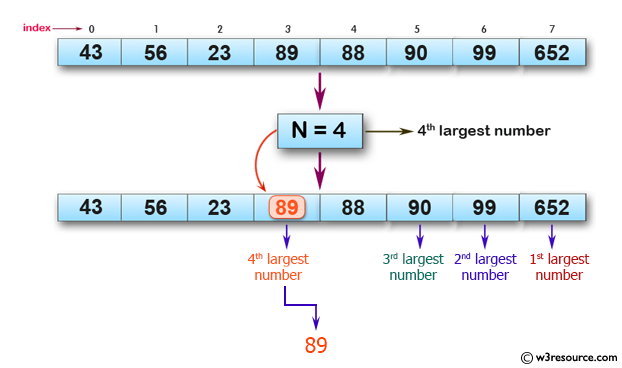
Sample Solution:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript function to get nth largest element from an unsorted array.</title>
</head>
<body>
</body>
</html>
JavaScript Code:
function nthlargest(arra,highest){
var x = 0,
y = 0,
z = 0,
temp = 0,
tnum = arra.length,
flag = false,
result = false;
while(x < tnum){
y = x + 1;
if(y < tnum){
for(z = y; z < tnum; z++){
if(arra[x] < arra[z]){
temp = arra[z];
arra[z] = arra[x];
arra[x] = temp;
flag = true;
}else{
continue;
}
}
}
if(flag){
flag = false;
}else{
x++;
if(x === highest){
result = true;
}
}
if(result){
break;
}
}
return (arra[(highest - 1)]);
}
console.log(nthlargest([ 43, 56, 23, 89, 88, 90, 99, 652], 4));
Sample Output:
89
Flowchart:
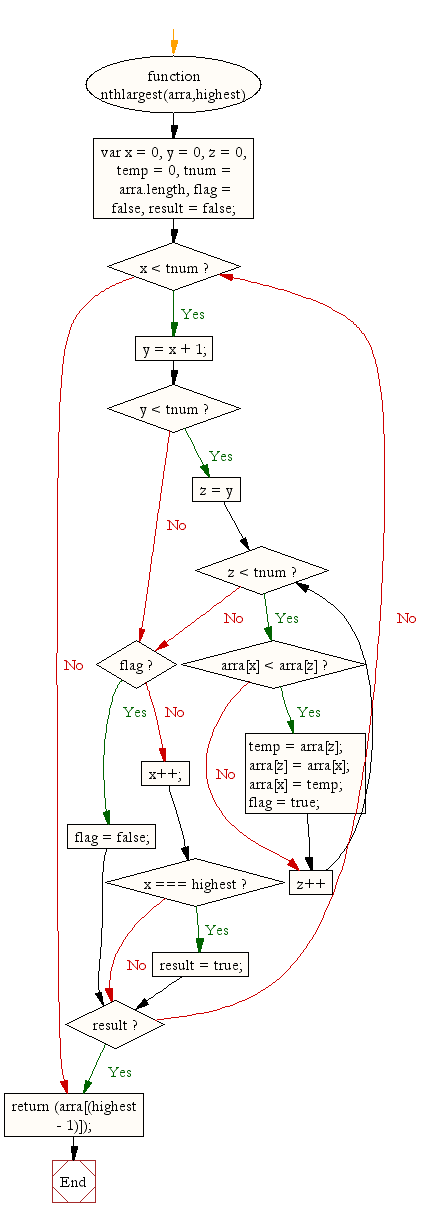
ES6 Version:
function nthlargest(arra,highest){
let x = 0;
let y = 0;
let z = 0;
let temp = 0;
const tnum = arra.length;
let flag = false;
let result = false;
while(x < tnum){
y = x + 1;
if(y < tnum){
for(z = y; z < tnum; z++){
if(arra[x] < arra[z]){
temp = arra[z];
arra[z] = arra[x];
arra[x] = temp;
flag = true;
}else{
continue;
}
}
}
if(flag){
flag = false;
}else{
x++;
if(x === highest){
result = true;
}
}
if(result){
break;
}
}
return (arra[(highest - 1)]);
}
console.log(nthlargest([ 43, 56, 23, 89, 88, 90, 99, 652], 4));
Live Demo:
See the Pen JavaScript - Get nth largest element from an unsorted array - array-ex- 34 by w3resource (@w3resource) on CodePen.
Improve this sample solution and post your code through Disqus
Previous: Write a JavaScript script to empty an array keeping the original.
Next: Write a JavaScript function to get a random item from an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
JavaScript: Tips of the Day
How to insert an item into an array at a specific index (JavaScript)?
What you want is the splice function on the native array object.
arr.splice(index, 0, item); will insert item into arr at the specified index (deleting 0 items first, that is, it's just an insert). In this example we will create an array and add an element to it into index 2:
var arr = []; arr[0] = "Jani"; arr[1] = "Hege"; arr[2] = "Stale"; arr[3] = "Kai Jim"; arr[4] = "Borge"; console.log(arr.join()); arr.splice(2, 0, "Lene"); console.log(arr.join());
Ref: https://bit.ly/2BXbp04
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework