Java String Exercises: Check whether the first two characters present at the end of a given string
Java String: Exercise-63 with Solution
Write a Java program to check whether the first two characters present at the end of a given string.
Sample Solution:
Java Code:
import java.util.*;
public class Main
{
public boolean firstInLast(String str)
{
if (str.length() < 2)
return false;
else if (str.substring(0,2).equals(str.substring(str.length()-2, str.length())))
return true;
else
return false;
}
public static void main (String[] args)
{
Main m= new Main();
String str1 = "educated";
System.out.println("The given strings is: "+str1);
System.out.println("The first two characters appear in the last is: "+m.firstInLast(str1));
}
}
Sample Output:
The given strings is: educated The first two characters appear in the last is: true
Pictorial Presentation:
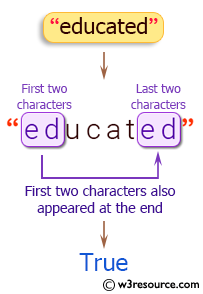
Flowchart:
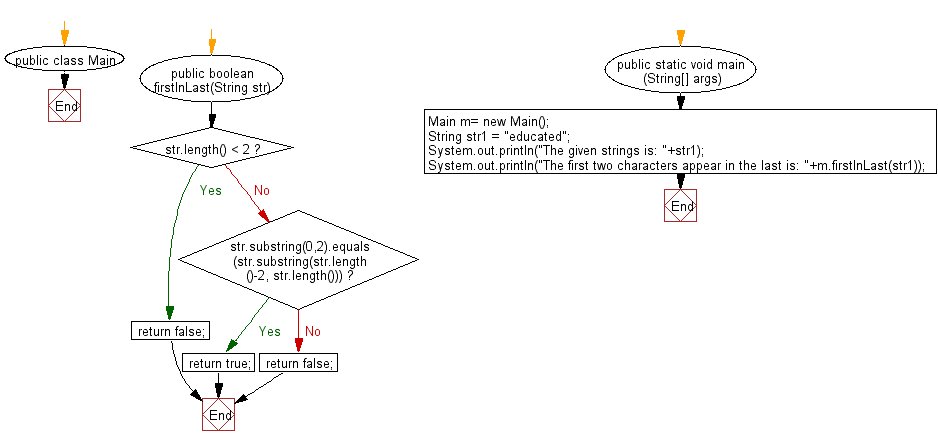
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to read a string and return true if "good" appears starting at index 0 or 1 in the given string.
Next: Write a Java program to read a string and if a substring of length two appears at both its beginning and end,return a string without the substring at the beginning otherwise, return the original string unchanged.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework