Java String Exercises: Check if two given strings are rotations of each other
Java String: Exercise-52 with Solution
Write a Java program to check if two given strings are rotations of each other.
Sample Solution:
Java Code:
import java.util.*;
class Main
{
static boolean checkForRotation (String str1, String str2)
{
return (str1.length() == str2.length()) && ((str1 + str1).indexOf(str2) != -1);
}
public static void main (String[] args)
{
String str1 = "ABACD";
String str2 = "CDABA";
System.out.println("The given strings are: "+str1+" and "+str2);
System.out.println("\nThe concatination of 1st string twice is: "+str1+str1);
if (checkForRotation(str1, str2))
{
System.out.println("The 2nd string "+str2+" exists in the new string.");
System.out.println("\nStrings are rotations of each other");
}
else
{
System.out.println("The 2nd string "+str2+" not exists in the new string.");
System.out.printf("\nStrings are not rotations of each other");
}
}
}
Sample Output:
The given strings are: ABACD and CDABA The concatination of 1st string twice is: ABACDABACD The 2nd string CDABA exists in the new string. Strings are rotations of each other
Pictorial Presentation:
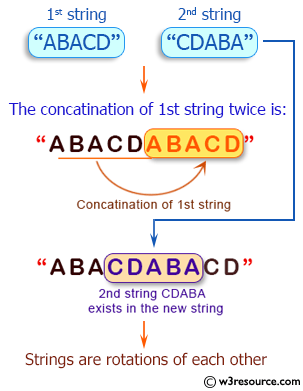
Flowchart:
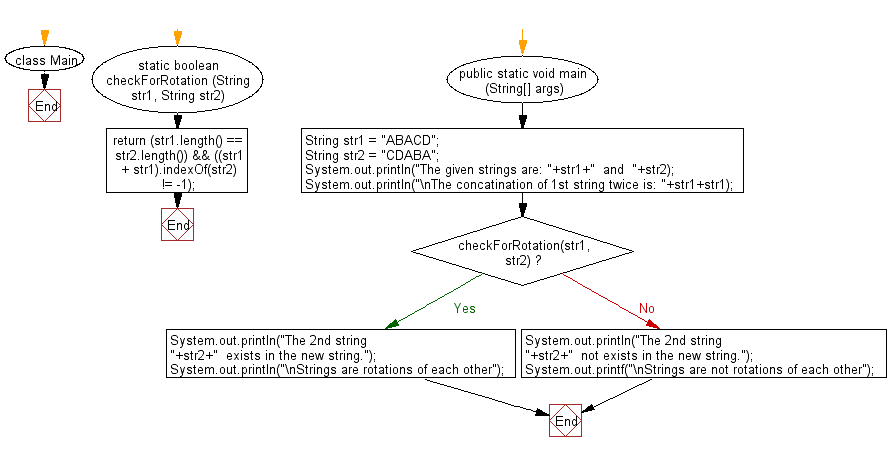
Visualize Java code execution (Python Tutor):
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to count and print all the duplicates in the input string.
Next: Write a Java program to match two strings where one string contains wildcard characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework