Java String Exercises: Find longest Palindromic Substring within a string
Java String: Exercise-32 with Solution
Write a Java program to find longest Palindromic Substring within a string.
Pictorial Presentation:
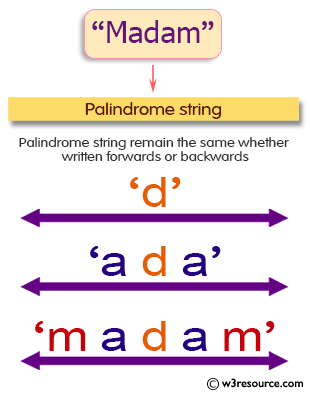
Sample Solution:
Java Code:
import java.util.*;
public class Main {
static void printSubStr(String str1, int l, int h) {
System.out.println(str1.substring(l, h + 1));
}
static int longPalSubstr(String str1) {
int n = str1.length();
boolean table[][] = new boolean[n][n];
int mLength = 1;
for (int i = 0; i < n; ++i)
table[i][i] = true;
int strt = 0;
for (int i = 0; i < n - 1; ++i) {
if (str1.charAt(i) == str1.charAt(i + 1)) {
table[i][i + 1] = true;
strt = i;
mLength = 2;
}
}
for (int k = 3; k <= n; ++k) {
for (int i = 0; i < n - k + 1; ++i) {
int j = i + k - 1;
if (table[i + 1][j - 1] && str1.charAt(i) == str1.charAt(j)) {
table[i][j] = true;
if (k > mLength) {
strt = i;
mLength = k;
}
}
}
}
System.out.print("The longest palindrome substring in the given string is; ");
printSubStr(str1, strt, strt + mLength - 1);
return mLength;
}
public static void main(String[] args) {
String str1 = "thequickbrownfoxxofnworbquickthe";
System.out.println("The given string is: " + str1);
System.out.println("The length of the palindromic substring is: " + longPalSubstr(str1));
}
}
Sample Output:
The given string is: thequickbrownfoxxofnworbquickthe The longest palindrome substring in the giv en string is; brownfoxxofnworb The length of the palindromic substring is: 16
Flowchart:
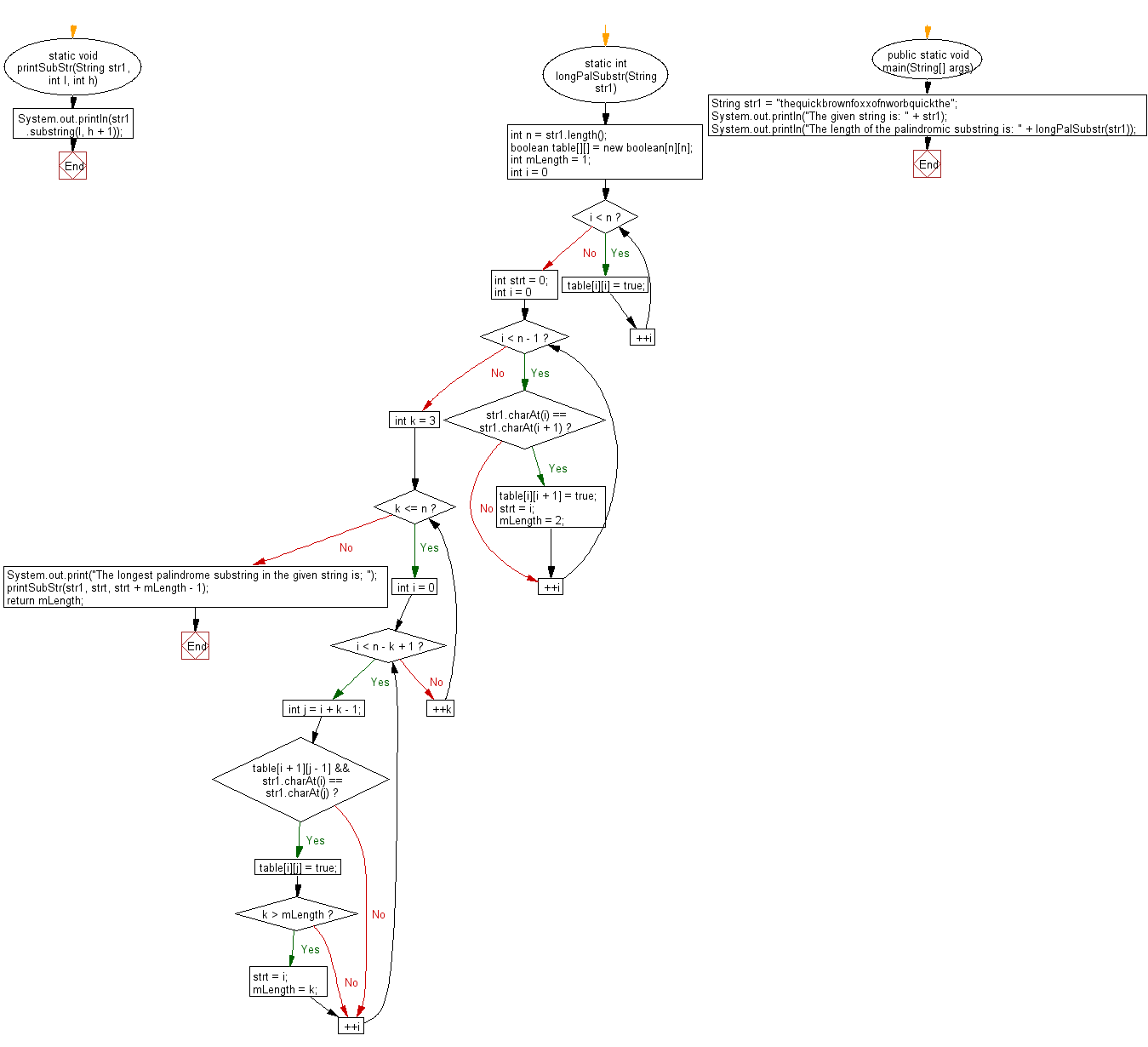
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a Java program to trim any leading or trailing whitespace from a given string.
Next: Write a Java program to find all interleavings of given strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework