Java Exercises: Check a number is a cyclic or not
Java Numbers: Exercise-19 with Solution
Write a Java program to check a number is a cyclic or not.
A cyclic number is an integer in which cyclic permutations of the digits are successive multiples of the number. The most widely known is 142857:
142857 × 1 = 142857
142857 × 2 = 285714
142857 × 3 = 428571
142857 × 4 = 571428
142857 × 5 = 714285
142857 × 6 = 857142
Input Data:
Input a number: 142857
Pictorial Presentation:
Sample Solution:
Java Code:
import java.util.Scanner;
import java.math.BigInteger;
public class Example19 {
public static void main( String args[] ){
Scanner sc = new Scanner( System.in );
System.out.print("Input a number: ");
String strnum = sc.nextLine().trim();
BigInteger n = new BigInteger(strnum);
int len = strnum.length()+1;
String str = String.valueOf(len);
BigInteger n1 = new BigInteger(str);
StringBuilder buf = new StringBuilder();
for(int i = 0 ; i < (len-1); i++) {
buf.append('9');
}
BigInteger total = new BigInteger(buf.toString());
if(n.multiply(n1).equals(total)) {
System.out.println("It is a cyclic number.");
}
else {
System.out.println("Not a cyclic number.");
}
}
}
Sample Output:
Input a number: 142857 It is a cyclic number.
Flowchart:
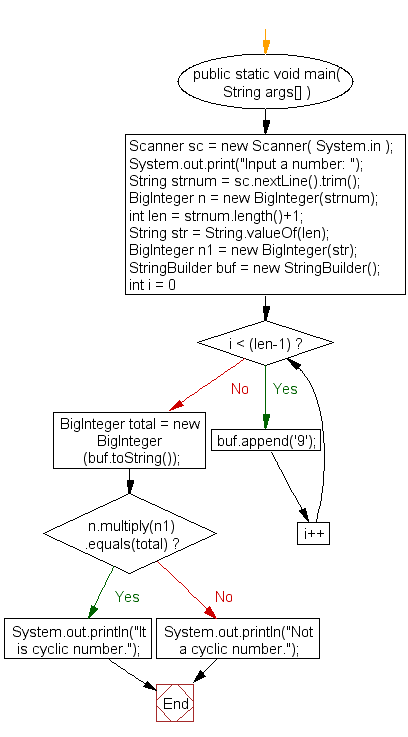
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to check a number is a cube or not.
Next: Write a Java program to display first 10 Fermat numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework