Java Math Exercises: Compute the result from the innermost brackets
Java Math Exercises: Exercise-23 with Solution
A fast scheme for evaluating a polynomial such as:
-19+ 7x- 4x2 + 6x3
when
x=3
is to arrange the computation as follows:((((0)x+6)x+(-4))x+7)x+(-19)
Write a Java program to compute the result from the innermost brackets.
Sample Solution:
Java Code:
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class solution {
public static void main(String[] args){
List<Double> coeffs = new ArrayList<Double>();
coeffs.add(-19.0);
coeffs.add(7.0);
coeffs.add(-4.0);
coeffs.add(6.0);
System.out.println(polyEval(coeffs, 3));
}
public static double polyEval(List<Double> coefficients, double x) {
Collections.reverse(coefficients);
Double accumulator = coefficients.get(0);
for (int i = 1; i < coefficients.size(); i++) {
accumulator = (accumulator * x) + (Double) coefficients.get(i);
}
return accumulator;
}
}
Ref.: https://bit.ly/3chhDot
Sample Output:
128.0
Flowchart:
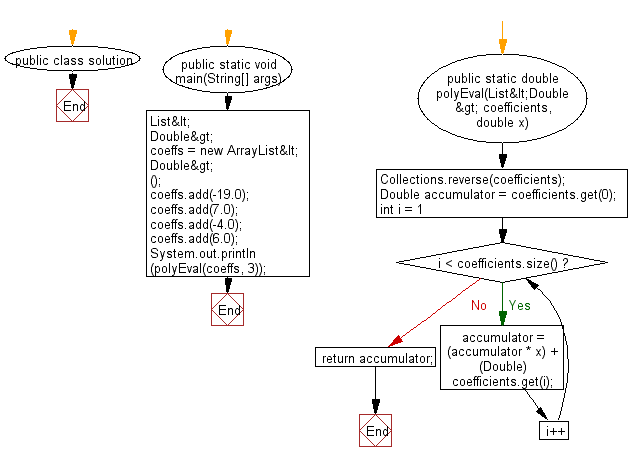
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to find next smallest palindrome.
Next: Write a Java program to calculate the Binomial Coefficient of two positive numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework