Java Conditional Statement Exercises: Displays the weekday between 1 and 7
Java Conditional Statement: Exercise-5 with Solution
Write a Java program that keeps a number from the user and generates an integer between 1 and 7 and displays the name of the weekday.
Test Data
Input number: 3
Pictorial Presentation:
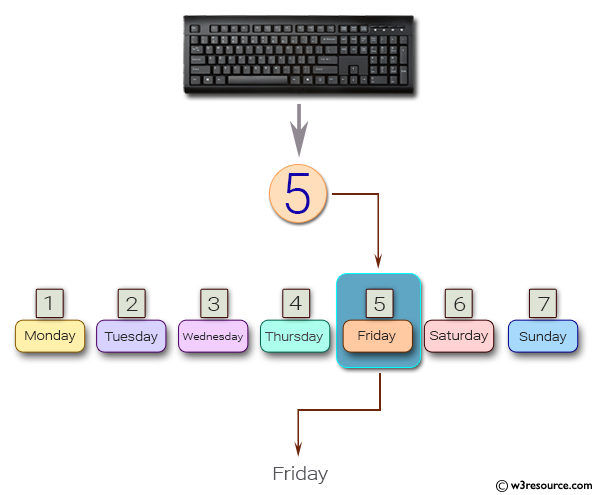
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise5 {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Input number: ");
int day = in.nextInt();
System.out.println(getDayName(day));
}
// Get the name for the Week
public static String getDayName(int day) {
String dayName = "";
switch (day) {
case 1: dayName = "Monday"; break;
case 2: dayName = "Tuesday"; break;
case 3: dayName = "Wednesday"; break;
case 4: dayName = "Thursday"; break;
case 5: dayName = "Friday"; break;
case 6: dayName = "Saturday"; break;
case 7: dayName = "Sunday"; break;
default:dayName = "Invalid day range";
}
return dayName;
}
}
Sample Output:
Input number: 3 Wednesday
Flowchart:
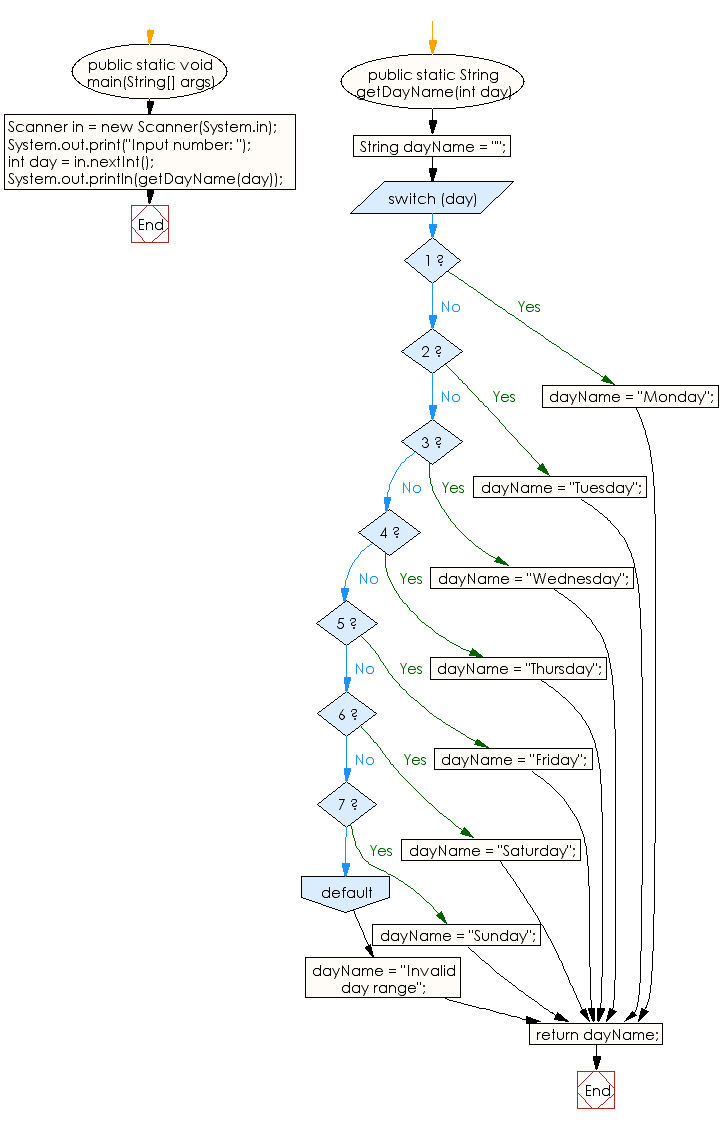
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program that reads a floating-point number and prints specified format.
Next: Write a Java program that reads in two floating-point numbers and tests whether they are the same up to three decimal places.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework