Java Conditional Statement Exercises: Solve quadratic equations
Java Conditional Statement: Exercise-2 with Solution
Write a Java program to solve quadratic equations (use if, else if and else).
Test Data
Input a: 1
Input b: 5
Input c: 1
Pictorial Presentation:
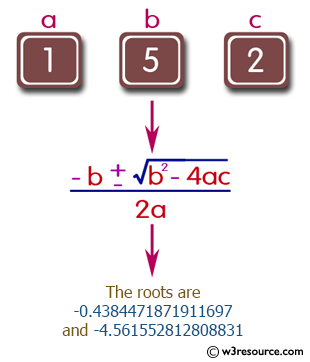
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise2 {
public static void main(String[] Strings) {
Scanner input = new Scanner(System.in);
System.out.print("Input a: ");
double a = input.nextDouble();
System.out.print("Input b: ");
double b = input.nextDouble();
System.out.print("Input c: ");
double c = input.nextDouble();
double result = b * b - 4.0 * a * c;
if (result > 0.0) {
double r1 = (-b + Math.pow(result, 0.5)) / (2.0 * a);
double r2 = (-b - Math.pow(result, 0.5)) / (2.0 * a);
System.out.println("The roots are " + r1 + " and " + r2);
} else if (result == 0.0) {
double r1 = -b / (2.0 * a);
System.out.println("The root is " + r1);
} else {
System.out.println("The equation has no real roots.");
}
}
}
Sample Output:
Input a: 1 Input b: 5 Input c: 2 The roots are -0.4384471871911697 and -4.561552812808831
Flowchart:
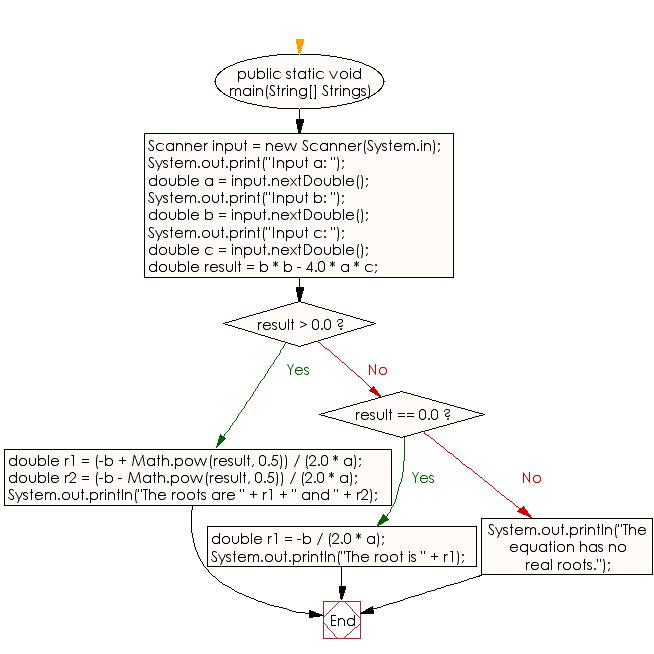
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to get a number from the user and print whether it is positive or negative.
Next: Write a Java program to to find the largest of three numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework