Java Collection, ArrayList Exercises: Replace the second element of a ArrayList with the specified element
Java Collection, ArrayList Exercises: Exercise-21 with Solution
Write a Java program to replace the second element of a ArrayList with the specified element.
Pictorial Presentation:
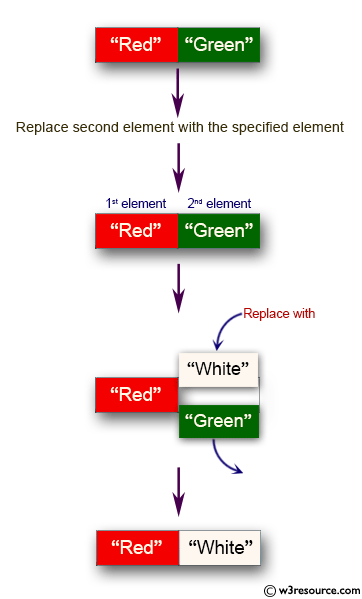
Sample Solution:-
Java Code:
import java.util.ArrayList;
public class Exercise21 {
public static void main(String[] args){
ArrayList<String> color = new ArrayList<String>();
color.add("Red");
color.add("Green");
System.out.println("Original array list: " + color);
String new_color = "White";
color.set(1,new_color);
int num=color.size();
System.out.println("Replace second element with 'White'.");
for(int i=0;i<num;i++)
System.out.println(color.get(i));
}
}
Sample Output:
Original array list: [Red, Green] Replace second element with 'White'. Red White
Flowchart:
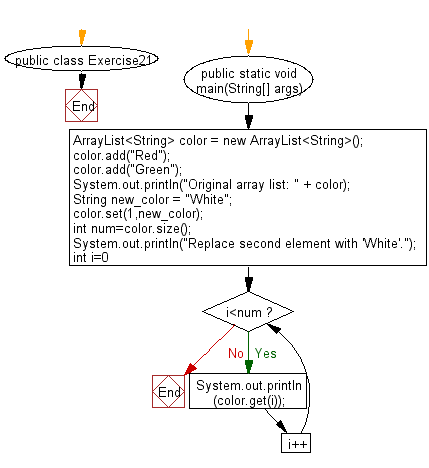
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Increase the size of an array list.
Next: Print all the elements of a ArrayList using the position of the elements.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework