Java Exercises: Check if the value 20 appears three times and no 20's are next to each other in a given array of integers
Java Basic: Exercise-98 with Solution
Write a Java program to check if the value 20 appears three times and no 20’s are next to each other in a given array of integers.
Pictorial Presentation:
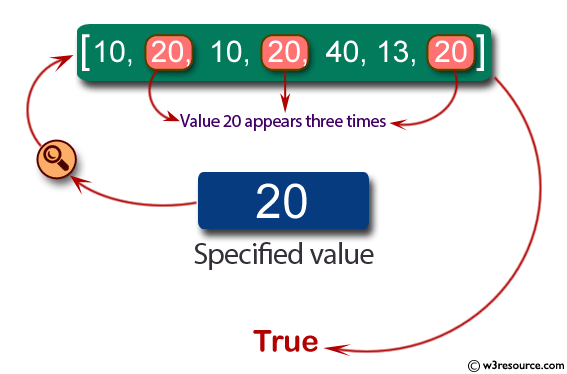
Sample Solution:
Java Code:
import java.util.*;
import java.io.*;
public class Exercise98 {
public static void main(String[] args)
{
int[] array_nums = {10, 20, 10, 20, 40, 13, 20};
int count = 0, result =0;
if(array_nums.length >= 1 && array_nums[0] == 20)
count++;
for(int i = 1; i < array_nums.length; i++) {
if(array_nums[i - 1] == 20 && array_nums[i] == 20)
{
System.out.printf( String.valueOf(false));
result = 1;
}
if(array_nums[i] == 20)
count++;
}
if (result==0)
{
System.out.printf( String.valueOf(count == 3));
}
System.out.printf("\n");
}
}
Sample Output:
true
Flowchart:
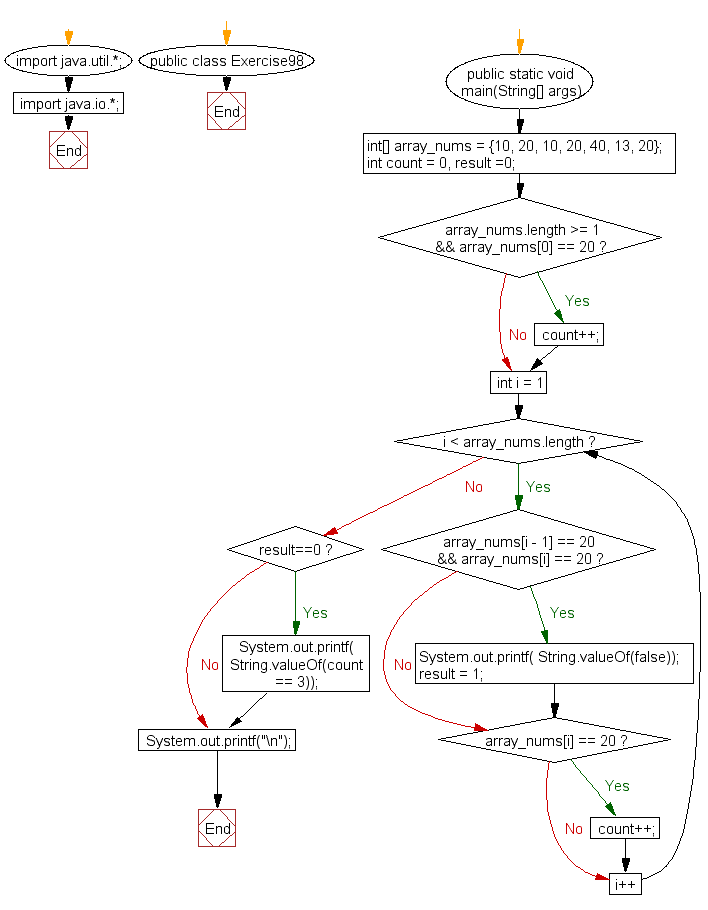
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to check if an array of integers contains a specified number next to each other or there are two same specified numbers separated by one element.
Next: Write a Java program to check if a specified number appears in every pair of adjacent element of a given array of integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework