Java Exercises: Create an array of string values
Java Basic: Exercise-95 with Solution
Write a Java program to create an array (length # 0) of string values. The elements will contain "0", "1", "2" … through ... n-1.
Pictorial Presentation:
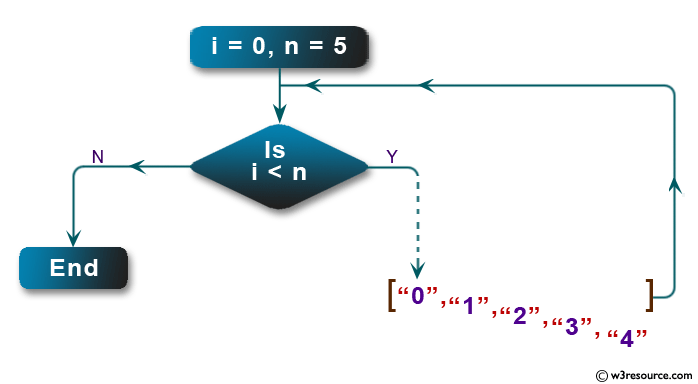
Sample Solution:
Java Code:
import java.util.*;
import java.io.*;
public class Exercise95 {
public static void main(String[] args)
{
int n= 5;
String[] arr_string = new String[n];
for(int i = 0; i < n; i++)
arr_string[i] = String.valueOf(i);
System.out.println("New Array: "+Arrays.toString(arr_string));
}
}
Sample Output:
New Array: [0, 1, 2, 3, 4]
Flowchart:

Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to rearrange all the elements of an given array of integers so that all the odd numbers come before all the even numbers.
Next: Write a Java program to check if there is a 10 in a given array of integers with a 20 somewhere later in the array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework