Java Exercises: Program than read an integer and calculate the sum of its digits and write the number of each digit of the sum in English
Java Basic: Exercise-87 with Solution
Write a Java program than read an integer and calculate the sum of its digits and write the number of each digit of the sum in English.
Sample Solution:
Java Code:
import java.io.*;
public class Main {
public static void main(String[] args) {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
try {
int sum = 0;
String str = br.readLine();
char[] numStr = str.toCharArray();
for (int i = 0; i < numStr.length; i ++) {
sum += numStr[i] - '0';
}
System.out.println("Original Number: "+str);
print_number(sum);
} catch (IOException e) {
e.printStackTrace();
}
}
public static void print_number(int n) {
int x; int y; int z;
String[] number = {"zero","one","two","three","four","five","six","seven","eight","nine"};
System.out.println("Sum of the digits of the said number: "+n);
if (n < 10) {
System.out.println(number[n]);
}
else if (n < 100) {
x = n / 10;
y = n - x *10;
System.out.println("In English: "+number[x] + " " + number[y]);
}
else {
x = n / 100;
y = (n - x * 100) / 10;
z = n - x * 100 - y * 10;
System.out.println("In English: "+number[x] + " " + number[y] + " " + number[z]);
}
}
}
If input 8
Sample Output:
Original Number: 8 Sum of the digits of the said number: 8 eight
Flowchart:
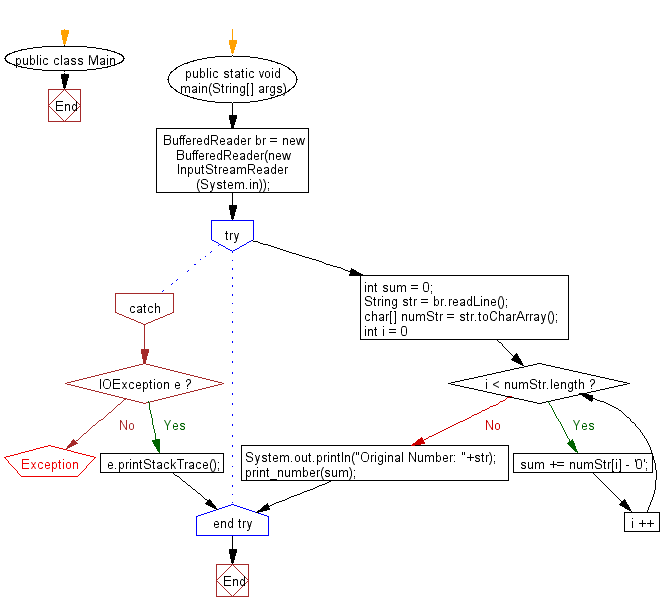
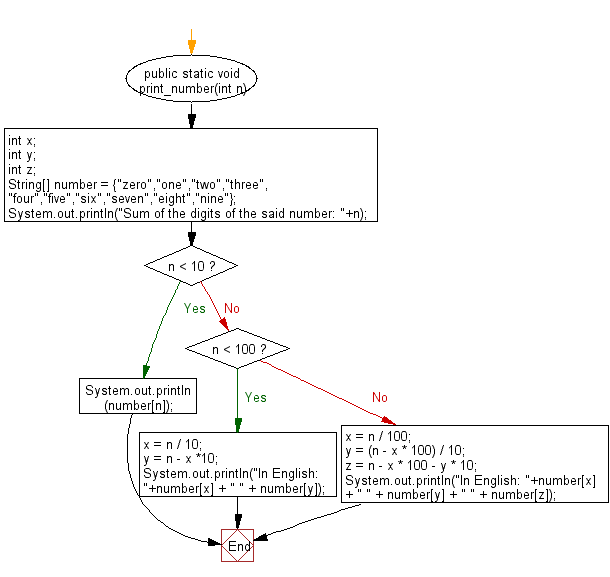
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program start with an integer n, divide n by 2 if n is even or multiply by 3 and add 1 if n is odd, repeat the process until n = 1.
Next: Write a Java program to get the current system environment and system properties.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework