Java Exercises: Create a new array of length 2 from two arrays of integers with three elements and the new array will contain the first and last elements from the two arrays
Java Basic: Exercise-77 with Solution
Write a Java program to create a new array of length 2 from two arrays of integers with three elements and the new array will contain the first and last elements from the two arrays.
Sample Solution:
Java Code:
import java.util.Arrays;
public class Exercise77 {
public static void main(String[] args)
{
int[] array1 = {50, -20, 0};
int[] array2 = {5, -50, 10};
System.out.println("Array1: "+Arrays.toString(array1));
System.out.println("Array2: "+Arrays.toString(array2));
int[] array_new = {array1[0], array2[2]};
System.out.println("New Array: "+Arrays.toString(array_new));
}
}
Sample Output:
Array1: [50, -20, 0] Array2: [5, -50, 10] New Array: [50, 10]
Pictorial Presentation:
Flowchart:
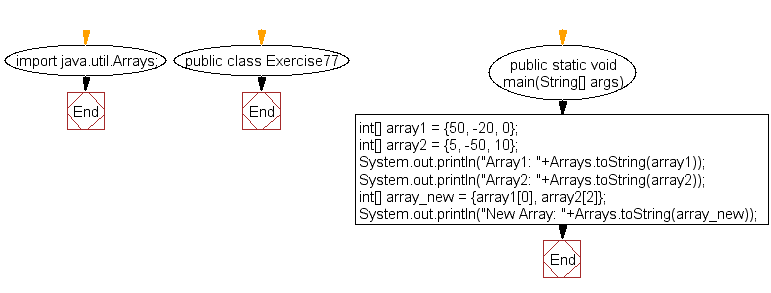
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to test if the first or the last elements of two arrays of integers are same.
Next: Write a Java program to test that a given array of integers of length 2 contains a 4 or a 7.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework