Java Exercises: Create a new string taking first three characters from a given string
Java Basic: Exercise-72 with Solution
Write a Java program to create a new string taking first three characters from a given string. If the length of the given string is less than 3 use "#" as substitute characters.
Test Data: str1 = "Python"
str2 = " "
Sample Solution:
Java Code:
import java.lang.*;
public class Exercise72 {
public static void main(String[] args)
{
String str1 = "";
int len = str1.length();
if(len >= 3)
System.out.println( str1.substring(0, 3));
else if(len == 1)
System.out.println( (str1.charAt(0)+"##"));
else
System.out.println("###");
}
}
Sample Output:
###
Pictorial Presentation:
Flowchart:
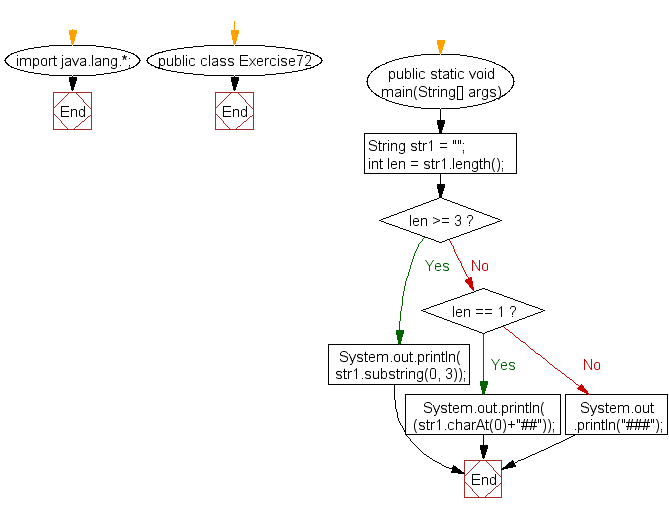
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to create the concatenation of the two strings except removing the first character of each string.
Next: Write a Java program to create a new string taking first and last characters from two given strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework