Java Exercises: Calculate the modules of two numbers without using any inbuilt modulus operator
Java Basic: Exercise-65 with Solution
Write a Java program to calculate the modules of two numbers without using any inbuilt modulus operator.
Test data:
Input the first number : 5
Input the second number: 3
2
Input the first number : 19
Input the second number: 7
5
Sample Solution:
Java Code:
import java.util.*;
public class Exercise65 {
public static void main(String[] args)
{
Scanner in = new Scanner(System.in);
System.out.print("Input the first number : ");
int a = in.nextInt();
System.out.print("Input the second number: ");
int b = in.nextInt();
int divided = a / b;
int result = a - (divided * b);
System.out.println(result);
}
}
Sample Output:
Input the first number : 19 Input the second number: 7 5
Flowchart:
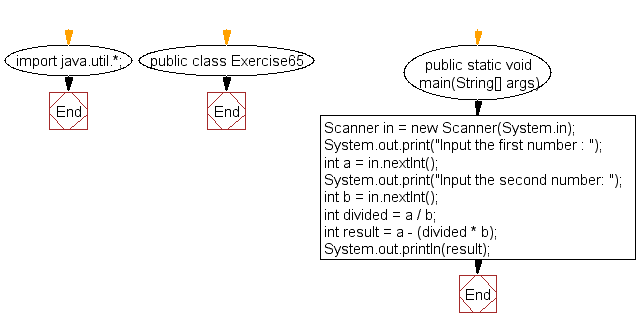
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program that accepts two integer values between 25 to 75 and return true if there is a common digit in both numbers.
Next:Write a Java program to compute the sum of the first 100 prime numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework