Java Exercises: Compute the area of a hexagon
Java Basic: Exercise-34 with Solution
Write a Java program to compute the area of a hexagon.
Test Data:
Input the length of a side of the hexagon: 6
Pictorial Presentation: Area of Hexagon
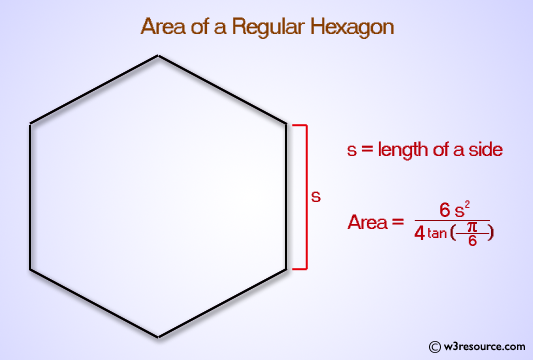
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise34 {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Input the length of a side of the hexagon: ");
double s = input.nextDouble();
System.out.print("The area of the hexagon is: " + hexagonArea(s)+"\n");
}
public static double hexagonArea(double s) {
return (6*(s*s))/(4*Math.tan(Math.PI/6));
}
}
Sample Output:
Input the length of a side of the hexagon: 6 The area of the hexagon is: 93.53074360871938
Flowchart:
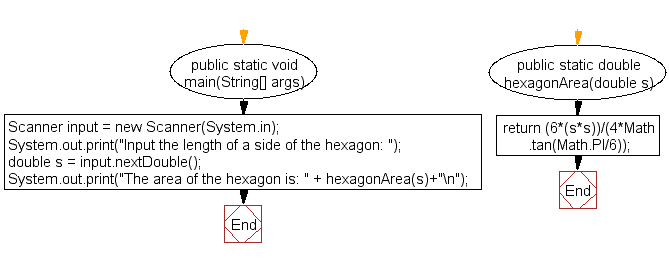
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program and compute the sum of the digits of an integer.
Next: Write a Java program to compute the area of a polygon.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework