Java Exercises: Convert a hexadecimal to a binary number
Java Basic: Exercise-29 with Solution
Write a Java program to convert a hexadecimal to a binary number.
Hexadecimal number: This is a positional numeral system with a radix, or base, of 16. Hexadecimal uses sixteen distinct symbols, most often the symbols 0-9 to represent values zero to nine, and A, B, C, D, E, F (or alternatively a, b, c, d, e, f) to represent values ten to fifteen.
Binary number: A binary number is a number expressed in the base-2 numeral system or binary numeral system. This system uses only two symbols: typically 1 (one) and 0 (zero).
Test Data:
Input any hexadecimal number: 37
Pictorial Presentation: Hexadecimal to Binary number
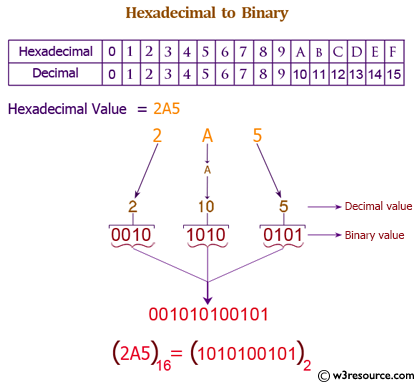
Sample Solution:
Java Code:
import java.util.Scanner;
public class Exercise29 {
public static int hex_to_decimal(String s)
{
String digits = "0123456789ABCDEF";
s = s.toUpperCase();
int val = 0;
for (int i = 0; i < s.length(); i++)
{
char c = s.charAt(i);
int d = digits.indexOf(c);
val = 16*val + d;
}
return val;
}
public static void main(String args[])
{
String hexdec_num;
int dec_num, i=1, j;
int bin_num[] = new int[100];
Scanner scan = new Scanner(System.in);
System.out.print("Enter Hexadecimal Number : ");
hexdec_num = scan.nextLine();
/* convert hexadecimal to decimal */
dec_num = hex_to_decimal(hexdec_num);
/* convert decimal to binary */
while(dec_num != 0)
{
bin_num[i++] = dec_num%2;
dec_num = dec_num/2;
}
System.out.print("Equivalent Binary Number is: ");
for(j=i-1; j>0; j--)
{
System.out.print(bin_num[j]);
}
System.out.print("\n");
}
}
Sample Output:
Enter Hexadecimal Number : 37 Equivalent Binary Number is: 110111
Flowchart:
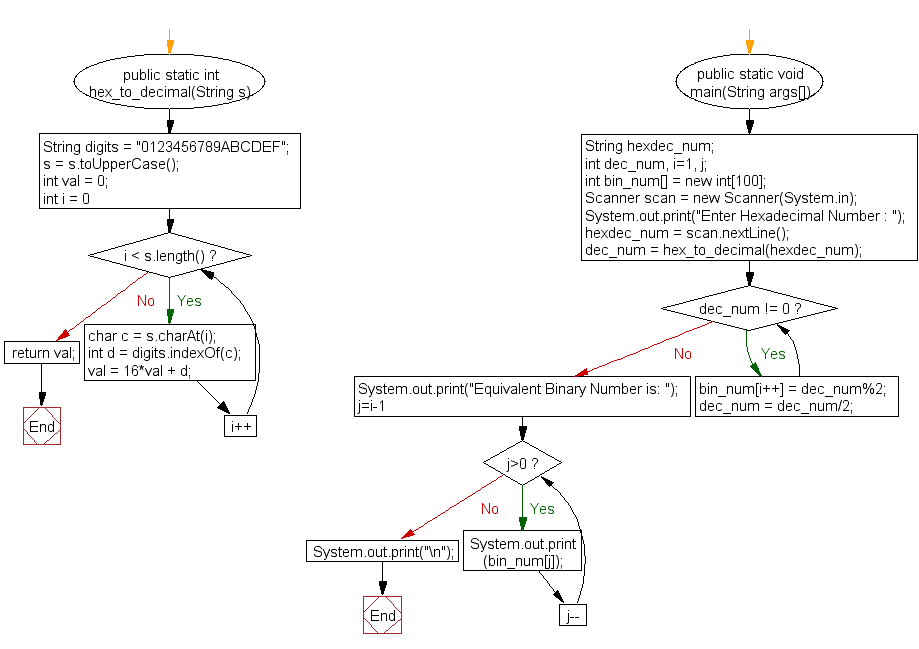
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to convert a hexadecimal to a decimal number.
Next: Write a Java program to convert a hexadecimal to a octal number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
Java: Tips of the Day
How to sort an ArrayList?
Collections.sort(testList); Collections.reverse(testList);
That will do what you want. Remember to import Collections though!
Ref: https://bit.ly/32urdSe
- New Content published on w3resource:
- HTML-CSS Practical: Exercises, Practice, Solution
- Java Regular Expression: Exercises, Practice, Solution
- Scala Programming Exercises, Practice, Solution
- Python Itertools exercises
- Python Numpy exercises
- Python GeoPy Package exercises
- Python Pandas exercises
- Python nltk exercises
- Python BeautifulSoup exercises
- Form Template
- Composer - PHP Package Manager
- PHPUnit - PHP Testing
- Laravel - PHP Framework
- Angular - JavaScript Framework
- Vue - JavaScript Framework
- Jest - JavaScript Testing Framework